20 вариантов использования ChatGPT в IT с примерами
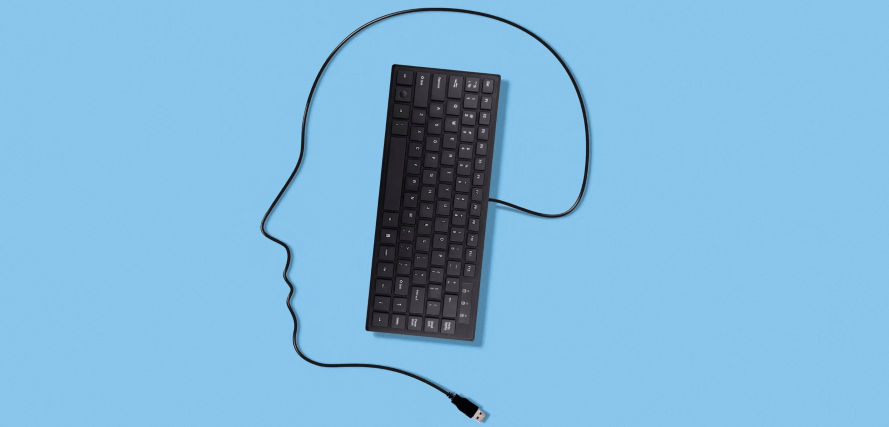
ChatGPT — это чат‑бот на основе нейросети, разработанной инженерами компании OpenAI. Его основные задачи состоят в поддержании диалога с пользователем и выдаче релевантной информации по запросу.
В этой статье говорим про использование ChatGPT в IT. От генерации кода, юнит тестов и front-end клиентов, до генерации CV, Job description'ов, скриптов видео роликов и многое другое.
Как следует читать эту статью? PS: не с помощью саммари от ChatGPT 😄
Статья поделена на пункты, в каждом пункте есть примеры с ➡️ input: команда которую я даю чат-боту и 🖨️ output: то, что мне отвечает ChatGPT.
Хотел бы отметить, что ChatGPT понимает множество языков, в том числе и русский, но для большей "нативности" ответов я использую английский язык.
Enjoy 🤓
1. 📜 Code snippets
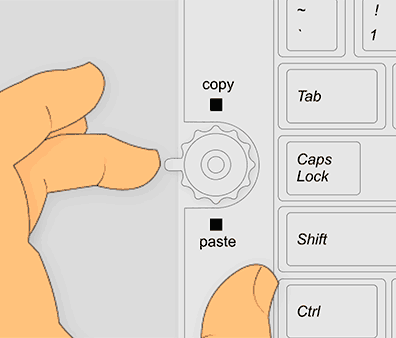
ChatGPT может быть обучен на примерах кода для генерации фрагментов кода или даже целых программ на различных языках программирования. Это может быть использовано для автоматизации некоторых рутинных задач, связанных с написанием кода, таких как написание шаблонного кода или даже готовых решений
Пример использования генерации кода в ChatGPT:
мой ➡️ input запрос:
i wanna to create a simple program in .NET language to make currency exchange calculator with real currency rate integrations
Вот что мне выдал ChatGPT как 🖨️ output:
Sure! Here's a simple example of how you can create a currency exchange calculator in .NET using the System.Net.Http namespace to retrieve the latest exchange rates from a free API:
using System;
using System.Net.Http;
using System.Threading.Tasks;
namespace CurrencyExchange
{
class Program
{
static void Main(string[] args)
{
Console.WriteLine("Enter the amount you want to convert: ");
string amountString = Console.ReadLine();
double amount = double.Parse(amountString);
Console.WriteLine("Enter the currency code of the currency you want to convert FROM: ");
string fromCurrency = Console.ReadLine();
Console.WriteLine("Enter the currency code of the currency you want to convert TO: ");
string toCurrency = Console.ReadLine();
double exchangeRate = GetExchangeRate(fromCurrency, toCurrency).Result;
double convertedAmount = exchangeRate * amount;
Console.WriteLine($"{amount} {fromCurrency} is equal to {convertedAmount} {toCurrency}");
}
static async Task<double> GetExchangeRate(string fromCurrency, string toCurrency)
{
string apiKey = "your-api-key-here";
string apiUrl = $"https://api.exchangerate-api.com/v4/latest/{fromCurrency}";
HttpClient client = new HttpClient();
client.DefaultRequestHeaders.Add("Authorization", apiKey);
HttpResponseMessage response = await client.GetAsync(apiUrl);
string result = await response.Content.ReadAsStringAsync();
// Parse the exchange rate from the JSON response
dynamic exchangeRates = JsonConvert.DeserializeObject(result);
double exchangeRate = exchangeRates.rates[toCurrency];
return exchangeRate;
}
}
}
Вуаля, и у меня есть программа которая умеет парсить валюты, что от меня нужно? Всего лишь получить apikey
и вставить его в сеттинги. Но это еще не все, следите за руками:
➡️ input: Я прошу его порефакторить код, который он только что сгенерил и он мне отвечает, что добавил Error handling'а и других фич
This version of the code includes additional input validation to ensure that the user enters a valid amount and currency code. It also converts the currency codes to uppercase to ensure that they match the format used by the exchange rate API.
и вот 🖨️ output: вторая версия порефактореного кода
using System;
using System.Net.Http;
using System.Threading.Tasks;
namespace CurrencyExchange
{
class Program
{
static void Main(string[] args)
{
// Get the amount to convert and the currency codes
Console.WriteLine("Enter the amount you want to convert: ");
double amount = GetInputAmount();
string fromCurrency = GetCurrencyCode("FROM");
string toCurrency = GetCurrencyCode("TO");
// Get the exchange rate and convert the amount
double exchangeRate = GetExchangeRate(fromCurrency, toCurrency).Result;
double convertedAmount = exchangeRate * amount;
Console.WriteLine($"{amount} {fromCurrency} is equal to {convertedAmount} {toCurrency}");
}
static async Task<double> GetExchangeRate(string fromCurrency, string toCurrency)
{
string apiKey = "your-api-key-here";
string apiUrl = $"https://api.exchangerate-api.com/v4/latest/{fromCurrency}";
HttpClient client = new HttpClient();
client.DefaultRequestHeaders.Add("Authorization", apiKey);
HttpResponseMessage response = await client.GetAsync(apiUrl);
string result = await response.Content.ReadAsStringAsync();
// Parse the exchange rate from the JSON response
dynamic exchangeRates = JsonConvert.DeserializeObject(result);
double exchangeRate = exchangeRates.rates[toCurrency];
return exchangeRate;
}
static double GetInputAmount()
{
double amount = 0;
bool validInput = false;
while (!validInput)
{
string amountString = Console.ReadLine();
if (double.TryParse(amountString, out amount))
{
validInput = true;
}
else
{
Console.WriteLine("Invalid input. Please enter a valid amount: ");
}
}
return amount;
}
static string GetCurrencyCode(string currencyType)
{
Console.WriteLine($"Enter the currency code of the currency you want to convert {currencyType}: ");
string currencyCode = Console.ReadLine().ToUpper();
// Validate the currency code
if (currencyCode.Length != 3)
{
Console.WriteLine("Invalid currency code. Please enter a valid 3-letter currency code: ");
return GetCurrencyCode(currencyType);
}
return currencyCode;
}
}
}
Разницу 2х версий можно просмотреть тут.
2. 🧪 Генератор юнит тестов
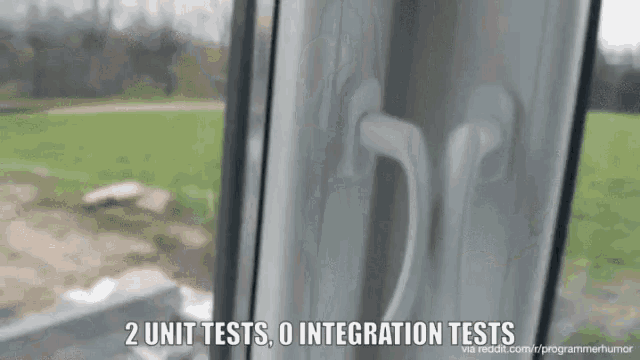
Весьма приятным бонусом есть то, что он может не только генерировать код, но и тесты к нему.
Пример генерации unit тестов с помощью ChatGPT
➡️ input: generate the unit tests for the last codebase
🖨️ output:
using System.Threading.Tasks;
using Xunit;
namespace CurrencyExchange.Tests
{
public class ProgramTests
{
[Theory]
[InlineData("USD", "EUR", 1.2)]
[InlineData("GBP", "JPY", 142.3)]
[InlineData("AUD", "CAD", 0.9)]
public async Task GetExchangeRate_ReturnsCorrectExchangeRate(string fromCurrency, string toCurrency, double expectedExchangeRate)
{
// Arrange
double actualExchangeRate;
// Act
actualExchangeRate = await Program.GetExchangeRate(fromCurrency, toCurrency);
// Assert
Assert.Equal(expectedExchangeRate, actualExchangeRate, 2);
}
[Fact]
public void GetInputAmount_ReturnsValidAmount()
{
// Arrange
double expectedAmount = 123.45;
// Act
TestConsole.SetInput("123.45");
double actualAmount = Program.GetInputAmount();
// Assert
Assert.Equal(expectedAmount, actualAmount, 2);
}
[Theory]
[InlineData("usd", "USD")]
[InlineData("GBP", "GBP")]
[InlineData("jpy", "JPY")]
public void GetCurrencyCode_ReturnsValidCurrencyCode(string input, string expectedCurrencyCode)
{
// Arrange
// Act
TestConsole.SetInput(input);
string actualCurrencyCode = Program.GetCurrencyCode("Test");
// Assert
Assert.Equal(expectedCurrencyCode, actualCurrencyCode);
}
}
}
3. 🧭Генерация API и front-end клиента
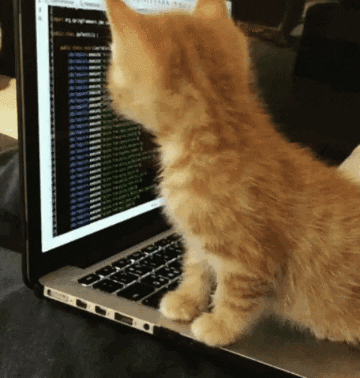
Далее просим ChatGPT сгенерировать вместо консольного приложения API.
Пример генерации API via ChatGPT
➡️ input: convert the refactored code base to Web API
🖨️ output:
using Microsoft.AspNetCore.Mvc;
using System.Threading.Tasks;
namespace CurrencyExchange.Controllers
{
[Route("api/[controller]")]
[ApiController]
public class CurrencyController : ControllerBase
{
[HttpGet("{fromCurrency}/{toCurrency}/{amount}")]
public async Task<IActionResult> Convert(string fromCurrency, string toCurrency, double amount)
{
double exchangeRate = await GetExchangeRate(fromCurrency, toCurrency);
double convertedAmount = exchangeRate * amount;
return Ok(new { amount, fromCurrency, toCurrency, exchangeRate, convertedAmount });
}
private async Task<double> GetExchangeRate(string fromCurrency, string toCurrency)
{
string apiKey = "your-api-key-here";
string apiUrl = $"https://api.exchangerate-api.com/v4/latest/{fromCurrency}";
HttpClient client = new HttpClient();
client.DefaultRequestHeaders.Add("Authorization", apiKey);
HttpResponseMessage response = await client.GetAsync(apiUrl);
string result = await response.Content.ReadAsStringAsync();
dynamic exchangeRates = JsonConvert.DeserializeObject(result);
double exchangeRate = exchangeRates.rates[toCurrency];
return exchangeRate;
}
}
}
Далее просим создать AI для нас фронт на react.js
Пример генерации front-end клиента API с помощью ChatGPT
➡️ input: please create a front-end for these api on react.js
🖨️ output:
import React, { useState } from 'react';
function CurrencyConverter() {
const [amount, setAmount] = useState(0);
const [fromCurrency, setFromCurrency] = useState("USD");
const [toCurrency, setToCurrency] = useState("EUR");
const [exchangeRate, setExchangeRate] = useState(0);
const [convertedAmount, setConvertedAmount] = useState(0);
const [error, setError] = useState(null);
async function handleSubmit(e) {
e.preventDefault();
try {
const response = await fetch(`/api/currency/${fromCurrency}/${toCurrency}/${amount}`);
const data = await response.json();
setExchangeRate(data.exchangeRate);
setConvertedAmount(data.convertedAmount);
} catch (err) {
setError(err.message);
}
}
return (
<form onSubmit={handleSubmit}>
<h1>Currency Converter</h1>
{error &amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;&amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp; <p className="error">{error}</p>}
<label htmlFor="amount">
Amount:
<input
type="number"
id="amount"
value={amount}
onChange={e => setAmount(e.target.value)}
/>
</label>
<label htmlFor="fromCurrency">
From Currency:
<input
type="text"
id="fromCurrency"
value={fromCurrency}
onChange={e => setFromCurrency(e.target.value)}
/>
</label>
<label htmlFor="toCurrency">
To Currency:
<input
type="text"
id="toCurrency"
value={toCurrency}
onChange={e => setToCurrency(e.target.value)}
/>
</label>
<button type="submit">Convert</button>
{exchangeRate &amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;&amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp; (
<p>
{amount} {fromCurrency} is equal to {convertedAmount} {toCurrency}
</p>
)}
</form>
);
}
export default CurrencyConverter;
Выглядит неплохо, особенно если не сильно разбираешься в react'e 😀. Теперь попросим еще сгенерировать стилей.
Пример генерации css в ChatGPT
➡️ input: please add some css styles to the latest example
🖨️ output js файла:
import './CurrencyConverter.css';
function CurrencyConverter() {
// ...
return (
<form className="currency-converter" onSubmit={handleSubmit}>
<h1>Currency Converter</h1>
{error &amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;&amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp; <p className="error">{error}</p>}
<label htmlFor="amount">
Amount:
<input
type="number"
id="amount"
value={amount}
onChange={e => setAmount(e.target.value)}
/>
</label>
<label htmlFor="fromCurrency">
From Currency:
<input
type="text"
id="fromCurrency"
value={fromCurrency}
onChange={e => setFromCurrency(e.target.value)}
/>
</label>
<label htmlFor="toCurrency">
To Currency:
<input
type="text"
id="toCurrency"
value={toCurrency}
onChange={e => setToCurrency(e.target.value)}
/>
</label>
<button type="submit">Convert</button>
{exchangeRate &amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;&amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp;amp; (
<p>
{amount} {fromCurrency} is equal to {convertedAmount} {toCurrency}
</p>
)}
</form>
);
}
🖨️ и аутпут css файла:
.currency-converter {
max-width: 600px;
margin: 0 auto;
padding: 20px;
border: 1px solid #ccc;
border-radius: 5px;
}
.currency-converter h1 {
text-align: center;
margin-bottom: 30px;
}
.currency-converter label {
display: block;
margin-bottom: 10px;
}
.currency-converter input {
padding: 10px;
border: 1px solid #ccc;
border-radius: 5px;
width: 100%;
box-sizing: border-box;
font-size: 16px;
}
.currency-converter button {
background-color: #4CAF50;
color: white;
padding: 14px 20px;
margin-top: 20px;
border: none;
border-radius: 4px;
cursor: pointer;
width: 100%;
}
.currency-converter p {
margin-top: 20px;
font-size: 18px;
}
.currency-converter .error {
color: red;
}
4. 🗎 Создание технической документации
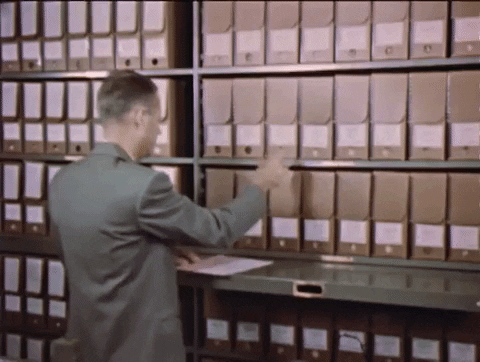
ChatGPT поможет генерировать техническую документацию . Вы можете сделать это в нужном вам формате предварительно обучив модель.
Пример создания технической документации в ChatGPT:
Продолжаем чат с пункта №1 и просим сгенерировать техническую документацию для нашего конвертора валют.
➡️ input: create a tech documentation for the last code
🖨️ output:
Overview
This program allows the user to enter an amount in one currency and convert it to another currency using the latest exchange rates from the "ExchangeRate-API" (https://www.exchangerate-api.com/).
Input
The program prompts the user to enter the following:
- The amount to convert. The program only accepts valid double numbers.
- The 3-letter currency code of the currency the amount will be converted from.
- The 3-letter currency code of the currency the amount will be converted to.
Output
The program displays the amount converted to the desired currency using the exchange rate obtained from the API.
API Key
In order to use the program, you need to sign up for a free API key from the ExchangeRate-API website and replace "your-api-key-here" in line 20 of the program.
Validation
The program includes additional input validation to ensure that the user enters a valid amount, and a 3-letter currency code. The program will keep prompting the user to enter a valid input until a valid input is provided.
How it works
- The program prompts the user to enter the amount, the currency code of the currency to convert from and the currency code of the currency to convert to.
- Then it calls the GetInputAmount() method to get a valid amount from the user, and the GetCurrencyCode() method to get a valid currency code from the user.
- Once a valid input is obtained, the program calls the GetExchangeRate() method to get the latest exchange rate from the API by passing the from and to currency code to the method.
- The program then performs the conversion using the obtained exchange rate, and display the result on the console.
Dependencies
This program uses the following .NET namespaces:
- System.Net.Http
- System.Threading.Tasks
It also uses a JSON deserializer such as Newtonsoft.Json to parse the response from the API.
5. 🗄️ Генерация тест кейсов и тестовых данных
ChatGPT может помочь и в рутинной генерации тест кейсов, давайте возьмем за пример user story 2 отсюда:
➡️ input:
User story: As a user, I want to be able to request the cash from my account at an ATM so that I will be able to receive the money from my account quickly and in different places.
Scenario 1: Requesting the cash from a creditworthy account
Given: that the account is creditworthy
And: the card is valid
And: the dispenser contains cash
When: the customer requests the cash
Then: ensure the account is debited
And: ensure cash is dispensed
And: ensure the card is returned
Scenario 2: Requesting the cash from an overdrawn account
Given: that the account is overdrawn
And: the card is valid
When: the customer requests the cash
Then: ensure the rejection message is displayed
And: ensure cash isn’t dispensed
далее просим сгенерировать тест кейсы и даем еще 1 ➡️ input: please generate the test cases for User story and scenario 1-2
и вот какой 🖨️ output получаем:
Sure, here are some test cases that could be used to test the functionality described in the user story and scenarios 1 and 2:
Scenario 1: Requesting the cash from a creditworthy account
Test Case 1:
- Input: Account balance = $500, Requested amount = $100
- Output: Account balance = $400, $100 cash dispensed, Card returned
- Expected Result: Cash is dispensed successfully and account balance is updated
Test Case 2:
- Input: Account balance = $100, Requested amount = $200
- Output: Account balance = $100, $0 cash dispensed, Card returned
- Expected Result: Requested amount is more than the account balance, cash is not dispensed and account balance remains the same
Test Case 3:
- Input: Account balance = $1000, Requested amount = $1000
- Output: Account balance = $0, $1000 cash dispensed, Card returned
- Expected Result: All the funds are withdrawn from the account and cash is dispensed successfully
Scenario 2: Requesting the cash from an overdrawn account
Test Case 1:
- Input: Account balance = -$100, Requested amount = $50
- Output: Rejection message displayed, $0 cash dispensed, Card returned
- Expected Result: Account is overdrawn and cash is not dispensed
Test Case 2:
- Input: Account balance = -$200, Requested amount = $100
- Output: Rejection message displayed, $0 cash dispensed, Card returned
- Expected Result: Account is overdrawn and cash is not dispensed
Test Case 3:
- Input: Account balance = -$500, Requested amount = $1000
- Output: Rejection message displayed, $0 cash dispensed, Card returned
- Expected Result: Account is overdrawn and cash is not dispensed
These test cases will help you check the basic functionality of the ATM system for creditworthy and overdrawn accounts. Additionally, you could also test it under different conditions like low or out of cash scenario on ATM, or multiple transactions from same account in a short time span.
Также можем попросить конвертнуть это все в json формат, и вот что получим на выходе от ChatGPT 🖨️:
{
"Scenario 1: Requesting the cash from a creditworthy account": [
{
"Test Case 1": {
"input": {
"Account balance": 500,
"Requested amount": 100
},
"output": {
"Account balance": 400,
"Cash dispensed": 100,
"Card returned": true
}
}
},
{
"Test Case 2": {
"input": {
"Account balance": 100,
"Requested amount": 200
},
"output": {
"Account balance": 100,
"Cash dispensed": 0,
"Card returned": true
}
}
},
{
"Test Case 3": {
"input": {
"Account balance": 1000,
"Requested amount": 1000
},
"output": {
"Account balance": 0,
"Cash dispensed": 1000,
"Card returned": true
}
}
}
]
}
{
"Scenario 2: Requesting the cash from an overdrawn account": [
{
"Test Case 1": {
"input": {
"Account balance": -100,
"Requested amount": 50
},
"output": {
"Rejection message displayed": true,
"Cash dispensed": 0,
"Card returned": true
}
}
},
{
"Test Case 2": {
"input": {
"Account balance": -200,
"Requested amount": 100
},
"output": {
"Rejection message displayed": true,
"Cash dispensed": 0,
"Card returned": true
}
}
},
{
"Test Case 3": {
"input": {
"Account balance": -500,
"Requested amount": 1000
},
"output": {
"Rejection message displayed": true,
"Cash dispensed": 0,
"Card returned": true
}
}
}
]
}
6. 🎫 Summary технических документов / статей / новостей
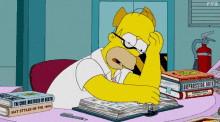
ChatGPT можно обучить извлекать ключевые моменты и обобщать технические документы, руководства, отчеты, исследовательские работы и т.д., делая информацию более доступной и быстрой для восприятия.
Пример summary тех. документации с помощью ChatGPT
В принципе, это работает не только на техничиских документах, но и на любых документах. Возьмем как пример страницу Reactivity in Depth в документации к vue.js ➡️ Please summarize in one paragraph
+ скопированый текст страницы, вот что я получил как результат 🖨️:
Vue's reactivity system is one of the framework's most distinctive features. It makes state management simple and intuitive. Reactivity is a programming paradigm that allows the adjustment of changes in a declarative manner, for example, when a cell value in an Excel spreadsheet is updated, it automagically updates other cells that depend on it. In JavaScript, it needs to be done manually by wrapping the code that updates the dependent variables in a function, making it a subscriber to its dependencies, and then detecting when a variable is mutated to notify all subscriber functions to re-run. In Vue, it's done by intercepting the reading and writing of object properties using getter/setters or Proxies.
пример summary новостей/статей
Если у вас нет времени читать новости, или статьи, но вы хотите понимать контекст, можно юзать ChatGPT
к примеру, берем новость и просим подсумировать ее и вот что получаем на выходе:
Displace, an upstart company, has showcased a completely wireless 55-inch 4K OLED TV that runs on four hot-swappable batteries at CES 2023. The TV can be linked up to four TVs together for a 110-inch 8K picture. The cost for a single Displace TV is $3,000, a four-TV bundle is expected to run $9,000, with the possibility of linking up to 16 Displace units for an enormous 220-inch 16K image.
7. 🔪 Генерация Job description для рекрутеров
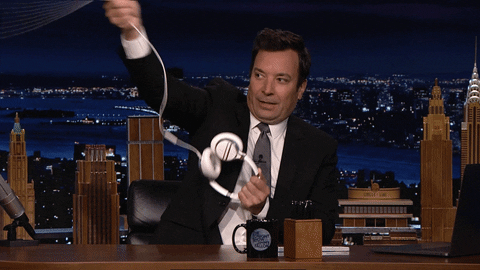
Типичная ситуация что у вас есть запрос от клиента или хайринг менеджера, что нужно получить, но вам лень генерить вручную или просто хотите упростить себе работу.
Пример генерации Job description с помощью ChatGPT
Мой инпут для ChatGPT ➡️:
Please generate a job description: we need an expert .NET engineer with Azure and .NET core experience. Mandatory the REDIS, and MongoDB. Also, we have a legacy part of the system with asp.net MVC and Oracle.
Вот что мне ответил ChatGPT 🖨️ :
Job Title: Expert .NET Engineer
Job Description:
We are seeking an experienced and highly skilled .NET Engineer to join our team. The ideal candidate will have a strong background in .NET development, as well as expertise in Azure and .NET Core. This is an excellent opportunity for a motivated individual to work on cutting-edge technology and make a significant impact on the development and success of our company.
Key Responsibilities:
- Develop, maintain, and improve software products and services using .NET, Azure, and .NET Core.
- Work on the development of new features, as well as maintenance and support of existing features.
- Collaborate with cross-functional teams to design and implement solutions that meet the needs of our customers.
- Ensure the performance, quality, and responsiveness of applications.
- Collaborate with other engineers and stakeholders to identify, troubleshoot, and resolve issues.
Required Skills and Experience:
- Strong experience with .NET development and Azure.
- Strong experience with .NET Core.
- Experience with REDIS and MongoDB
- Experience with asp.net MVC and Oracle is a plus.
- Strong understanding of software development principles and design patterns.
- Experience with Agile development methodologies.
- Excellent problem-solving, debugging, and troubleshooting skills.
- Strong written and verbal communication skills.
Education and Training:
- Bachelor's degree in Computer Science, Computer Engineering, or a related field.
- Minimum of 5 years of experience in software development.
Our company offers a competitive salary, comprehensive benefits, and a dynamic work environment. If you are a motivated and experienced .NET Engineer, we would like to hear from you. Please apply today!
Выглядит весьма сносно 😊
8. 🤠Генерация CV и cover letter'ов
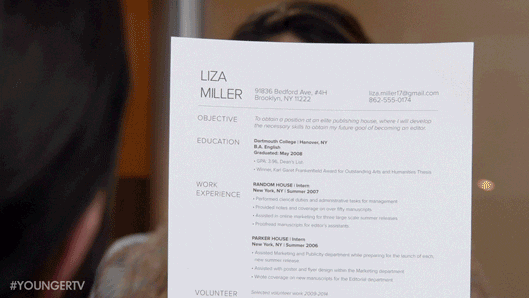
Если можно генерировать JD, то почему нельзя генерировать CV и cover letter-ы? 😉
Пример генерации Cover letter:
➡️ input: Please generate cover letter for follow job:
+ вставляю JD, например отсюда.
🖨️ output:
Dear Hiring Manager,
I am writing to express my interest in the Solutions Architect III position with Amazon.com Services LLC in Seattle, WA. My Master's degree in Computer Science and experience in Security Architecture, DevSecOps, Security Engineering, and Security Operations, I believe my qualifications align perfectly with the requirements of the role.
I have experience designing, building and operating large scale IT security systems both on-premises and in the cloud, I have gained extensive knowledge in cloud computing security, compliance, and risk management best practices. Additionally, as an AWS Certified Solutions Architect Associate, I have experience in AWS infrastructure and services and have supported security control implementation for commercial or US government compliance regimes.
Furthermore, my expertise in AWS cloud-native security architectures and best practices, such as AWS Well-Architected and Cloud Adoption Framework (CAF) has been honed in my experience working in a regulated industry such as financial services, healthcare and technology, where I have developed and shared program/project process frameworks, tools, and best practices that can be adopted throughout the organization.
In this role, I am excited to work with Amazon Distribution and Fulfillment Solutions (ADFS) service teams to convey cloud computing security, compliance, and risk management best practices, as well as aligning ADFS security implementation with broader enterprise standards and initiatives. Additionally, I am excited about the opportunity to develop technical content, such as security best practices, training material and reference architectures that can be used by ADFS to assist them in deploying secure and compliant AWS-based solutions.
Thank you for considering my application. I am eager to bring my skills and experience to Amazon and contribute to the success of the ADFS SCPT (Security, Compliance, Privacy, Trust) team. I am looking forward to the opportunity to speak with you further about my qualifications and how I can contribute to Amazon's success.
Please find my resume attached for your review.
Thank you for your time and consideration.
Sincerely,
[Your Name]
Вышло весьма неплохо, учитывая насколько напряжно обычно писать cover leter)
Пример генерации CV
Намерено даю минимум инфо, чтобы посмотреть как он справиться.
мой ➡️ инпут:
Please generate a CV based on the following experience:
Name: Artem Polishchuk
.NET stack - 10 years
Azure - 6 years
AWS - 2 years
People management for more than 5 years
Solution Architecture
also has hands-on experience with Java, angular, Xamarin
🖨️ output:
Name: Artem Polishchuk
Contact Information:
- Phone number:
- Email address:
- LinkedIn profile:
Summary: A highly skilled software developer with over 10 years of experience in the .NET stack, 6 years of experience in Azure, and 2 years of experience in AWS. Proven track record of success in people management and solution architecture, as well as hands-on experience with Java, Angular, and Xamarin. Committed to delivering high-quality solutions that meet the needs of customers and stakeholders.
Professional Experience:
Software Developer, XYZ Company (Date - Present)
- Developed and maintained software using the .NET stack and Azure
- Lead a team of developers in the implementation of new features and solutions
- Collaborated with stakeholders to understand requirements and ensure solutions met their needs
- Provided guidance and mentorship to junior team members
- Created solution architecture designs that balanced technical feasibility with business needs
Software Developer, ABC Inc. (Date - Date)
- Developed and maintained software using the .NET stack, Java, and Angular
- Worked on projects using Xamarin to create cross-platform mobile apps
- Collaborated with a team of developers to deliver solutions on time and on budget
- Participated in code reviews to ensure code quality and maintainability
- Helped to improve team productivity through implementation of agile methodologies
Technical Skills:
- .NET stack (10 years)
- Azure (6 years)
- AWS (2 years)
- Java
- Angular
- Xamarin
- Solution Architecture
- People Management (5+ years)
Education:
- Degree in Computer Science, University of XYZ (Date - Date)
Certifications:
- Microsoft Certified: Azure Developer Associate
- AWS Certified Developer - Associate
Awards & Recognitions
- Excellent Employee award on (Year)
- Recognition for outstanding performance in delivering projects on time and budget.
Languages:
- English (Fluent)
- Russian (Native)
Note: You need to fill in your own contact information, education, certifications and awards, date of employment, company name, languages you speak.
довольно большой кусок он сгенерировал, по сути готовый шаблон резюме, если ему скормить больше релевантной инфы, он сгенерит еще лучше результат.
9. 🗪 Сравнение двух разных текстов
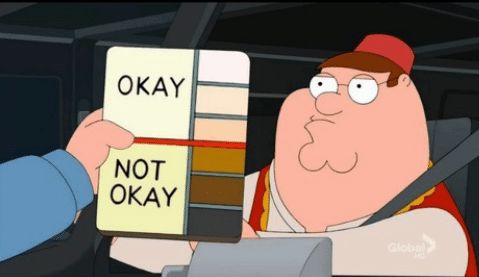
Теперь давайте сравним JD, который мы сгенерировали ранее и CV с примера №8:
➡️ input: Job description: + весь JD с примера №7
далее еще 1 ➡️ input:
please match our job description and CV: + CV с примера 8.
Вот какой 🖨️ output мы получили:
Artem Polishchuk's resume seems to match the job description for a .NET Engineer quite well. He has over 10 years of experience in the .NET stack, 6 years of experience in Azure, and 2 years of experience in AWS, which aligns with the job posting's requirements for strong experience with .NET development and Azure.
In his professional experience, Artem has demonstrated a track record of success in people management and solution architecture. He has also listed experience with Java, Angular, and Xamarin, which are listed as a plus in the job posting. Additionally, his experience collaborating with stakeholders and junior team members, as well as his ability to create solution architecture designs that balance technical feasibility with business needs, align with the responsibilities listed in the job posting.
He has listed the certifications of Microsoft Certified: Azure Developer Associate and AWS Certified Developer - Associate, which further demonstrates his expertise in Azure and AWS, as well as a solid understanding of cloud technology.
His education, a degree in Computer Science from the University of XYZ and awards & recognitions demonstrate his commitment to his profession and skill as a developer.
The only missing information is the contact information that is provided in the job posting. Otherwise his resume aligns very well with the job requirements.
Но работает это не всегда хорошо, например, берем пример резюме отсюда как ➡️ input:
Job description: + скопированый текст резюме с ссылки
и получаем 🖨️ output:
Alessandra Champlin's CV seems to match the job description for a .NET Engineer quite well. She has experience working as part of a team to develop a framework for the web-based mobile platform, customizing IBM Case Manager solutions and developing, documenting, and testing case management solutions using IBM Case Manager and IBM FileNet P8.
In her professional experience, she has demonstrated a strong experience with IBM Case Manager, IBM FileNet P8, Java, and has experience with people management. Additionally, her experience contributing to business development and proposal work, as well as developing client relationships aligns with the responsibilities listed in the job posting.
She has also experience working with micro-services teams on RESTful API designs and experience with Agile development environment.
Her education is in Computer Science and it shows her strong knowledge of JS and various framework such as React, Express, Node, JQuery, and others. Additionally, skills like XML/XSLT, good communication skills, JIRA, and knowledge of iOS Push notification behavior are also quite useful in this job.
One missing information from the resume is the required experience with Azure and .NET core which is mentioned in the job description.
Otherwise, her CV seems to be a strong match for the job requirements.
но мы можем и поспорить с чатом) ➡️ input:
why alessandra matched if she dont have a .net experience.
и что нам отвечает 🖨️:
I apologize for any confusion - you are correct that the job posting is specifically looking for an experienced .NET Engineer and requires experience with .NET development, Azure, and .NET Core, and Alessandra does not specifically list those technologies in her CV.
From the provided resume, it seems that Alessandra has primarily worked with IBM Case Manager, IBM FileNet P8, and Java, and while these technologies are not directly related to .NET, her experience in customizing case management solutions and working with developers, designers, and stakeholders to understand requirements and deliver solutions, may be transferable to similar work in a .NET environment.
However, given the specific requirement mentioned in the job posting, she may not have direct experience with the technologies mentioned on the job description.
But her experience, knowledge, skills and education make her suitable for this job, she just need to have hands-on experience with .NET and Azure or at least be willing to learn.
Т.е мы можем юзать этот инструмент чтобы делать какой-то прелиминари чек, но доверять на 100% его матчингу мы не можем.
10. ✔️Генерация Acceptance критериев
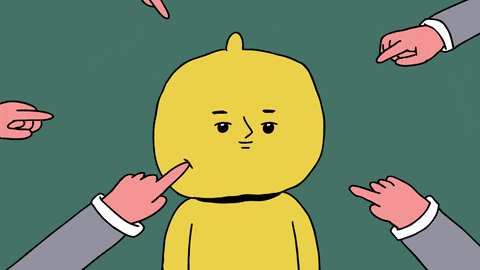
Окей, а что если у нас нет AC и мы хотим сгенерить их для какой-то фичи? ChatGPT и это нам поможет сделать:
➡️ input:
We have a cashback system where we store the customer data such as first name, last name, emails, clicks to offers, and history of purchases, and balance. We need to implement epic with GDPR compliance. Could you generate stories and acceptance criteria
🖨️ output:
Sure, here are a few user stories and acceptance criteria for implementing GDPR compliance in a cashback system:
Story 1: Customers should be able to request access to their personal data
- As a customer, I want to be able to request access to all the personal data that is stored about me, so that I can verify that it is correct and request changes if necessary.
- Acceptance Criteria:
- A customer can initiate a request for access to their personal data through a form on the website or mobile app.
- The request is sent to the data protection officer (DPO) or another designated person for processing.
- The DPO or designated person verifies the customer's identity and processes the request in a timely manner (e.g. within 30 days).
- The customer receives a copy of all the personal data that is stored about them in a format that is easy to read and understand.
Story 2: Customers should be able to request deletion of their personal data
- As a customer, I want to be able to request that my personal data be deleted from the system, so that I can protect my privacy.
- Acceptance Criteria:
- A customer can initiate a request for deletion of their personal data through a form on the website or mobile app.
- The request is sent to the DPO or another designated person for processing.
- The DPO or designated person verifies the customer's identity and processes the request in a timely manner (e.g. within 30 days).
- All personal data related to the customer is securely deleted from the system, and all backups are also erased.
Story 3: Customers should be able to request data portability
- As a customer, I want to be able to download a copy of my personal data in a standard format so that I can share it with other companies
- Acceptance Criteria:
- A customer can initiate a request for data portability through a form on the website or mobile app
- The request is sent to the DPO or another designated person for processing.
- The DPO or designated person verifies the customer's identity and processes the request in a timely manner (e.g. within 30 days).
- The customer receives a copy of all the personal data that is stored about them in a standard format (eg. CSV, JSON) that can be easily shared with other companies
Story 4: Regular Data Protection Impact Assessment (DPIA)
- As a data controller, We need to perform regular Data Protection Impact Assessment (DPIA) to understand the data protection risk and take the necessary action to mitigate them.
- Acceptance Criteria:
- We have a process for regular DPIA, that is performed periodically (e.g. annually)
- All the data controller and DPO role have the understanding on how to perform the DPIA.
- We have a process in place to mitigate identified risk from the DPIA.
Note that these stories and acceptance criteria are meant to be a starting point and may need to be tailored to your specific system and use case. Also, GDPR compliance is a large subject and this is just few of the aspect, it is advisable to consult with the legal expert or GDPR consultant to ensure full compliance.
11. 🔍Ответы на технические вопросы
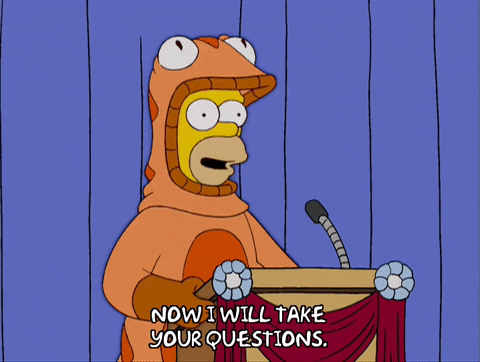
Вы можете юзать ChatGPT как Google. Отличие от Google в том, что он дает единый ответ, который вы можете перегенерировать. Но в отличие от гугла вам не нужно прыгать по статьям, он вам сразу дает выдержку.
Пример использования ChatGPT для ответов на ваши вопросы:
➡️ input: Explain me how the CDN works in simple words
🖨️ output:
A Content Delivery Network (CDN) is a system of distributed servers that deliver web content to users based on their geographic location. The goal of a CDN is to serve content to end-users with high availability and high performance.
When a user requests a web page, the request is sent to the nearest server in the CDN, which then delivers the requested content to the user. This is done by copying the content to multiple servers in different locations, and then routing user requests to the server that is closest to them. This helps to reduce the distance that data has to travel, and therefore improves the speed at which the content is delivered to the user.
In addition to improving performance, CDNs also help to improve availability by distributing content across multiple servers. If one server goes down, the CDN can route traffic to another server, so the content is still available to users.
Overall, a CDN is a way to speed up the delivery of content on a website by caching it on servers that are closer to the user, and providing multiple failover options if one server goes down.
теперь спросим кто лучший CDN провайдер:
➡️ input: the best cdn provider
🖨️ output:
There are many Content Delivery Network (CDN) providers to choose from, and the best one for you will depend on your specific needs and budget. Some of the most popular CDN providers include:
- Akamai: One of the largest and most well-established CDN providers. Akamai has a global network of servers and is known for its high performance and reliability.
- Cloudflare: A popular provider that offers both a CDN and a web application firewall (WAF). Cloudflare's network is distributed across 200+ cities in 90 countries, with a high focus on security and performance.
- Amazon Web Services (AWS): AWS offers a variety of services, including a CDN called Amazon CloudFront. CloudFront is integrated with other AWS services, making it easy to deliver content from an S3 bucket or an EC2 instance.
- Fastly: A edge cloud platform that provides a global network of servers and a high level of customization. They provide a number of advanced caching and security features, suitable for high traffic and big brands
- StackPath: They offer a global network of servers, with a focus on security and performance optimization. They have a wide range of enterprise-level services, such as DDoS protection and real-time analytics.
These are just a few examples, and there are many other providers to choose from as well. It's important to evaluate the features, performance and pricing of different providers to find the one that best meets your needs.
12. 💬 Генерация ответов для юзеров
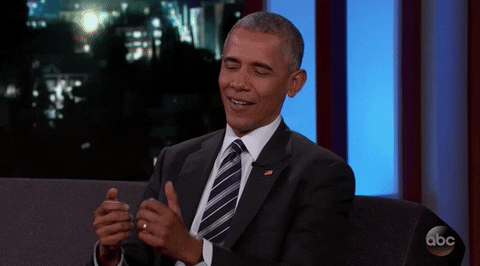
ChatGPT может юзаться для ответов на письма или использование совместно с чат ботом. Он может поддерживать диалог + может быть заточен под большое количество usecases, тк он понимает множество языков.
Обратите внимание на privacy, возможно ваша компания запрещает юзать подобные сервисы для анализа конфиденциальных писем.
13.🌐 Перевод на другие языки
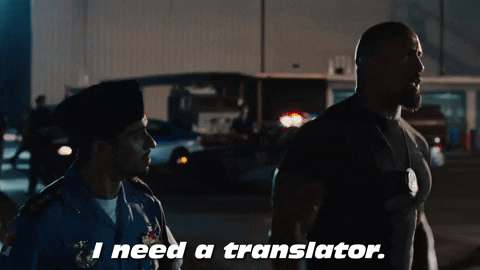
ChatGPT может юзаться как переводчик на другие языки. К примеру, мы можем перевести текст для локализации в react.js,
Пример локализации сайта с помощью ChatGPT
Берем простой пример c документации реакта по локализации и просим перевести на несколько языков:
➡️ input:
Here is code with text on English.
{
"title": "Welcome to react using react-i18next",
"description": {
"part1": "To get started, edit <1>src/App.js</1> and save to reload.",
"part2": "Switch language between english and german using buttons above."
}
}
i need the create a translates for localizations on follow languages:
russian
german
spain
🖨️ output:
{ "title": { "en": "Welcome to react using react-i18next", "de": "Willkommen bei React mit React-i18next", "ru": "Добро пожаловать в React с использованием React-i18next", "es": "Bienvenido a React usando react-i18next" }, "description": { "part1": { "en": "To get started, edit <1>src/App.js</1> and save to reload.", "de": "Um loszulegen, bearbeiten Sie <1>src/App.js</1> und speichern Sie die Änderungen.", "ru": "Чтобы начать, отредактируйте <1>src/App.js</1> и сохраните изменения.", "es": "Para empezar, edite <1>src/App.js</1> y guarde para recargar." }, "part2": { "en": "Switch language between english and german using buttons above.", "de": "Wechseln Sie die Sprache zwischen Englisch und Deutsch mit den obigen Schaltflächen.", "ru": "Переключите язык между английским и немецким с помощью кнопок выше.", "es": "Cambie el idioma entre inglés y alemán usando los botones de arriba." } } }
Note that in this example, I used the ISO 639-1 codes for the language ("en" for English, "de" for German, "ru" for Russian, "es" for Spanish) as the keys for the translations. This is a common convention for specifying translations in JSON.
Also, you'll need to use the appropriate library or tool that use this to translate your text (ex. react-i18next)
Please let me know if you have any other question or anything is not clear.
как видим он не понял сначала что мне нужно это все в разных файлах, даем ему еще один➡️ input:
no, i need to did it in separate files for each language
и он уже выдает коректный 🖨️ output:
Sure, you can keep the translations for each language in separate files. One common way to do this is to use the ISO 639-1 code for the language as the file name, with the ".json" file extension.
Here's an example of how your file structure might look like:
translations/
en.json
de.json
ru.json
es.json
Here's what the contents of
en.json
would look like:
{
"title": "Welcome to react using react-i18next",
"description": {
"part1": "To get started, edit <1>src/App.js</1> and save to reload.",
"part2": "Switch language between english and german using buttons above."
}
}
And the contents of
de.json
would look like:
{
"title": "Willkommen bei React mit React-i18next",
"description": {
"part1": "Um loszulegen, bearbeiten Sie <1>src/App.js</1> und speichern Sie die Änderungen.",
"part2": "Wechseln Sie die Sprache zwischen Englisch und Deutsch mit den obigen Schaltflächen."
}
}
here's what the contents of
ru.json
might look like:
{
"title": "Добро пожаловать в React с использованием React-i18next",
"description": {
"part1": "Чтобы начать, отредактируйте <1>src/App.js</1> и сохраните изменения.",
"part2": "Переключите язык между английским и немецким с помощью кнопок выше."
}
}
And here's what the contents of
es.json
might look like:
{
"title": "Bienvenido a React usando react-i18next",
"description": {
"part1": "Para empezar, edite <1>src/App.js</1> y guarde para recargar.",
"part2": "Cambie el idioma entre inglés y alemán usando los botones de arriba."
}
}
14. 🪓 Генерация описаний и ключевых слов
ChatGPT так же можно юзать для генерации описаний и ключевых слов к статьям:
➡️ input:
Сгенерируй мне СЕО описание и ключевые слова для названия статьи: 20 вариантов использования ChatGPT в IT с примерами
🖨️ output:
"20 практических примеров использования ChatGPT в IT: от автоматической генерации кода до создания искусственного интеллекта. ChatGPT – один из наиболее мощных инструментов для работы с текстом и искусственным интеллектом, который может быть использован в различных сферах бизнеса и индустрии. Мы расскажем о 20 реальных случаях его использования в IT и дадим конкретные примеры. "
Keywords: ChatGPT, IT, автоматическая генерация кода, искусственный интеллект, текстовая генерация, использование в бизнесе, использование в индустрии
15. 🎓 Обучение
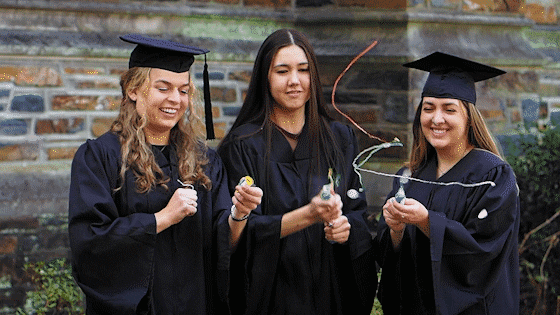
ChatGPT можно использовать для обучения. Он отлично генерирует программу обучения.
Пример:
Вы можете попросить сгенерировать roadmap для обучения MongoDB.
➡️ input:
i wanna learn MongoDB, please generate the roadmap for me
🖨️ output:
Sure! Here's a suggested roadmap for learning MongoDB:
- First, familiarize yourself with the basics of MongoDB and its architecture. Learn about the MongoDB data model, which uses documents and collections instead of tables and rows, and how this differs from traditional relational databases.
- Next, learn how to install and set up MongoDB on your system. You'll need to choose a version of MongoDB that is compatible with your operating system, and then install and configure it.
- Once you have MongoDB up and running, start exploring the MongoDB command-line interface (CLI). The CLI is a powerful tool that allows you to interact with MongoDB directly, and is essential for performing basic tasks such as creating and modifying databases and collections.
- After you've become comfortable with the CLI, start learning how to interact with MongoDB using one of the official MongoDB drivers for your preferred programming language. Some popular choices include the MongoDB drivers for Node.js, Python, and Java.
- Once you know how to connect to a MongoDB database and perform basic CRUD operations, you can start learning more advanced features such as indexes, aggregation, and database administration.
- Practice on mongodb with real world database such as shopping cart, social media database, ecommerce, etc.
- Practice with complex queries and check the performance of your queries, learn how to optimise them and identify potential bottlenecks.
- Look at MongoDB's built-in scalability and high availability options, including replica sets and sharded clusters, to understand how to scale MongoDB to meet the needs of your application.
- Join MongoDB community and stay updated with the latest features and best practices, and connect with other MongoDB users to get help and share your experiences.
Keep in mind that MongoDB is a powerful tool, and mastering it will take time and practice. But by following this roadmap and continually practicing and learning, you should be able to become proficient with MongoDB in no time!
16. 🖊️ Создание сценариев для видео или интерактивных туториалов
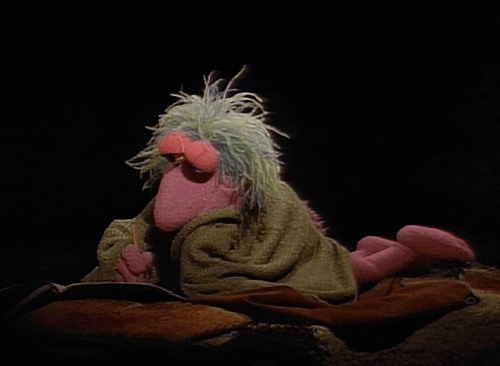
➡️ input:
please generate a script for a video that explain CDN
🖨️ output:
Sure, here's a script for a video that explains what a Content Delivery Network (CDN) is and how it works:
[Opening shot of a website loading on a computer screen]
Narrator: "When you visit a website, your computer needs to retrieve files from a server in order to display the site on your screen. But what happens when the server is located far away from you?"
[Map of the world appears on screen, with a red dot representing the location of the server and blue dots representing the locations of users trying to access the website]
Narrator: "If the server is located far away, it can take a long time for your computer to retrieve the files it needs. This can lead to slow loading times and a poor user experience."
[Diagram of a simple network appears on screen]
Narrator: "This is where a Content Delivery Network, or CDN, comes in. A CDN is a network of servers located around the world, each of which stores a copy of the files from a website."
[Map of the world appears again, this time with multiple red dots representing the locations of CDN servers]
Narrator: "When a user visits a website that uses a CDN, their request is directed to the closest CDN server. This means that the files are delivered to the user from a server that is geographically closer to them, which results in faster loading times."
[Diagram of the CDN network appears on screen, with arrows showing the flow of data from the server to the user]
Narrator: "In addition to improving loading times, CDNs can also help to reduce the load on the original server. By distributing the files across multiple servers, the CDN can handle a large number of requests without putting too much strain on any one server."
[Shot of a website loading quickly on a computer screen]
Narrator: "So the next time you visit a website and it loads quickly, it's likely that a CDN was used to make that happen. It's an simple, yet powerful way to improve the user experience for your website visitors.
[Closing shot of the logo or website of the company or service providing the CDN]
Narrator: "Thank you for watching and learning about CDN."
17. 🏢 Создание Meeting minutes, и самари разговоров
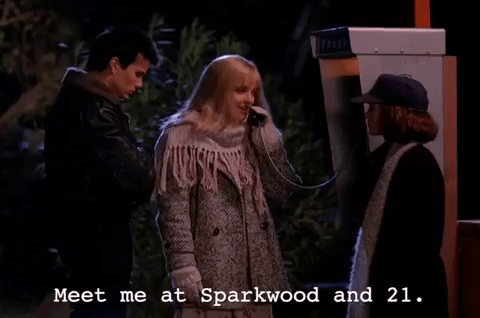
Часто бывает, когда многочасовой миттинг или большой емейл требует самари или создания action мейла,
Пример генерации meeting minutes с помощью ChatGPT
Возьмем пример скрипта разговора отсюда как ➡️ input:
please create meeting minutes + текст с pdf.
и вот какой 🖨️ output получаем:
Meeting Minutes:
City Council Meeting Date: (insert date) Location: Olathe City Hall
Call to Order: The Mayor called the meeting to order and asked everyone to say the Pledge of Allegiance.
Pledge of Allegiance: The Pledge of Allegiance was recited.
Special Business: -Council Member #1 presented a special award to the 3rd grade teachers of (insert school name here) Elementary School for their dedication and commitment to the students and community. The City Manager presented the teachers with a Certificate of Appreciation. -Council Member #2 thanked the students for their participation in the meeting and had the Assistant City Managers read a special proclamation. The teachers were presented with the proclamation.
Skate Park Proposal: The City Council discussed the proposal to build a new Skate park in the city to give young people a place to participate in activities such as skate boarding and extreme biking. The potential sites for the park were identified as Black Bob Park, Prairie Center Park, and Frontier Park. The council discussed the opinions of different groups of people throughout the city regarding the proposal.
Vote on Skate Park Proposal: The City Council voted on the proposal to build a new Skate park. The vote was (insert vote outcome).
Adjournment: The meeting was adjourned by the Mayor.
Submitted by: (insert your name)
Note: this is the skeleton of the meeting minutes , the specific details, vote outcome, name of the school, and other specific information needs to be filled according to your mock city council meeting.
18.❓ Создание quiz
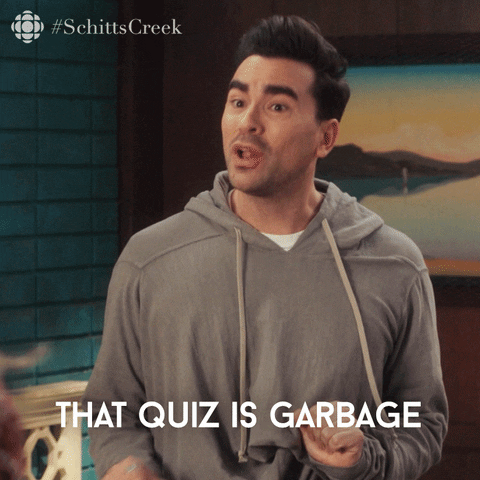
Вы можете попросить ChatGPT создать вам квиз на любую тему, например для javascript developers:
➡️ input: please generate me a quiz for javascript developers
🖨️ output:
Which of the following is not a JavaScript data type?
A) String
B) Boolean
C) Integer
D) Picture
What is the correct way to write a JavaScript array?
A) var colors = "red", "green", "blue";
B) var colors = ["red", "green", "blue"];
C) var colors = "red" "green" "blue";
D) var colors = "red, green, blue";
Which of the following is not a JavaScript loop?
A) for
B) while
C) do-while
D) repeat
What is the correct way to write a JavaScript function?
A) function myFunction()
B) function: myFunction()
C) myFunction()
D) myFunction: function()
What does the 'this' keyword refer to in JavaScript?
A) The current function
B) The global object
C) The object that the function is a property of
D) The object that the function is invoked on
What is the correct way to write a JavaScript conditional statement?
A) if x = y
B) if x == y
C) if x = y then
D) if x == y then
What does parseInt() function do?
A) Parses a string as a floating point number
B) Parses a string as an integer
C) Parses an integer as a string
D) Parses a floating point number as an integer
What is the difference between == and ===?
A) == compares values without considering data types while === compares values considering the data types.
B) == compares values considering the data types while === compares values without considering the data types
C) == and === do the same thing
D) == and === are not related to each other
How you can add an element to the end of an array
A) array.end(newItem)
B) array.push(newItem)
C) array[array.length] = newItem
D) array.add(newItem)
How you can remove the first item of an array?
A) array.remove(0)
B) array.pop()
C) array.shift()
D) array.start()
19. 💡 Помощь в улучшении продукта и генерации новых фич
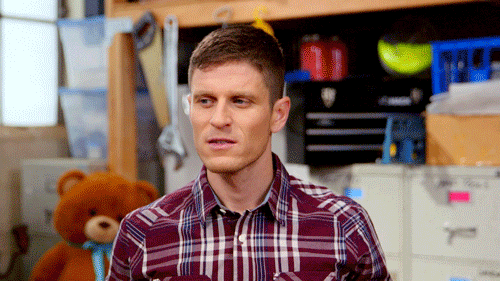
➡️ input:
We have a payment system that is integrated with two banks acquired. Also, online shops use our system as a payment method. which other features can we implement to increase revenue
🖨️ output:
There are several ways you can potentially increase revenue from your payment system, some possibilities include:
Adding new payment methods: By supporting additional payment methods, such as mobile wallets or alternative payment methods like Venmo or PayPal, you can attract more customers who prefer those options.
Offer recurring payments: Allowing customers to set up recurring payments for subscription-based services can increase revenue by making it easier for customers to maintain their subscriptions.
Implementing fraud detection and prevention: As you process more transactions, you may become a target for fraud. By implementing robust fraud detection and prevention measures, you can help ensure that your system remains secure and your customers' financial information is protected, which can improve customer trust and increase revenue over time.
Add loyalty program: You can consider implementing a loyalty program that rewards customers for using your payment system. This can encourage repeat business and also increase the amount of money customers spend on each transaction.
Add more value-added services: One of the main value-added services you can offer is faster payment and settlement. You could also consider offering a cash advance to your customers so that they can pay for goods and services faster.
Market more effectively : if your payment system is new, you could increase revenue by doing more marketing efforts, to make more merchants and customers aware of the system.
Increase fees: you may also increase revenue by raising the fees you charge for processing transactions.
You should consider which of these options would be the most feasible and beneficial for your business, and weigh the costs and benefits of each option before implementing any changes.
Also, it's important to be able to track the progress of your revenue growth and the impact of any changes you make to your payment system, so make sure to have the right tool to monitor your revenue and track your metrics.
20. 💻 Создание презентаций и статей
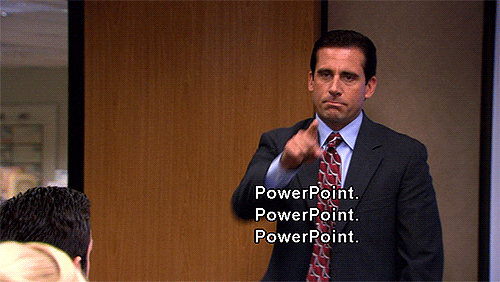
Когда вам нужно сгенерировать план презентации или статью, вы так же это можете сделать с помощью ChatGPT.
Пример создания PowerPoint презентации с помощью ChatGPT:
Он умеет работать только с текстовыми форматами, поэтому фото-контент и саму презентацию вам нужно будет сгенерировать самим.
➡️ input:
please generate me the script of PowerPoint presentation the company services.
our services are:
SEO optimization
Recruitment
website development
mobile app development
web design
🖨️ output:
Slide 1: Title slide
- Title: XYZ Company Services
- Subtitle: Helping businesses succeed in the digital world
Slide 2: SEO optimization
- Title: SEO Optimization
- Image: Picture of a search engine or a website
- Text:
- Search engine optimization, or SEO, is the process of improving the visibility of a website or a web page in a search engine's organic results.
- At XYZ Company, we use the latest techniques and best practices to help our clients improve their search engine rankings and attract more visitors to their websites.
- Whether you're looking to increase your visibility on Google, Bing or other search engines, we can help.
Slide 3: Recruitment
- Title: Recruitment Services
- Image: Picture of group of people in a meeting
- Text:
- At XYZ Company, we understand the importance of having the right team in place to drive your business forward.
- That's why we offer recruitment services to help you find the top talent you need to take your company to the next level.
- Our experienced recruiters can help you identify the best candidates for your open positions and support you through the hiring process.
Slide 4: Website Development
- Title: Website Development
- Image: Picture of a website
- Text:
- At XYZ Company, we offer website development services to help you create an effective online presence for your business.
- Our experienced developers can create a website that meets your needs and exceeds your expectations, using the latest technologies to ensure that your website is fast, secure, and easy to use.
- Whether you need a simple brochure website or a complex e-commerce platform, we can help.
Slide 5: Mobile App Development
- Title: Mobile App Development
- Image: Picture of mobile phone
- Text:
- As the popularity of smartphones and tablets continue to grow, mobile apps are becoming increasingly important for businesses of all sizes.
- At XYZ Company, we offer mobile app development services to help you create an app that is tailored to your specific needs and integrated with your existing systems.
- Our team of developers can create an app for you that is fast, easy to use and provide great User experience.
Slide 6: Web Design
- Title: Web Design
- Image: Picture of a website
- Text:
- The design of your website can be a powerful tool for attracting visitors and building trust.
- At XYZ Company, we offer web design services to help you create a website that is visually appealing and easy to navigate, while also being optimized for search engines and mobile devices.
- Our team of designers will work with you to create a design that reflects your brand and meets your specific requirements.
Slide 7: Conclusion
- Title: Thank you
- Text:
- Thank you for considering XYZ Company for your digital solutions.
- We hope you found this presentation informative and we look forward to working with you to achieve your business objectives.
- If you have any question, please don't hesitate to contact us.
Summary
Стоит учитывать вопрос privacy. Не всегда вы сможете загрузить скрипт разговора или рассказать достаточно детально об продукте из-за NDA или других ограничений, но в целом это довольно мощный инструмент. Он не сделает вашу работу за вас, но точно облегчит ее и ускорит решение поставленных задач. Поможет сгенерировать новые идеи или посмотреть на задачу под другим углом.
Также стоит помнить, что это все таки бот, хоть и довольно круто натренированный. ChatGPT не понимает что такое "вода" в тексте или "машинная речь", что оптимально, а что нет, следовательно вам нужно вычитывать и редактировать тексты которые он генерирует.