How to generate architecture diagrams with ChatGPT
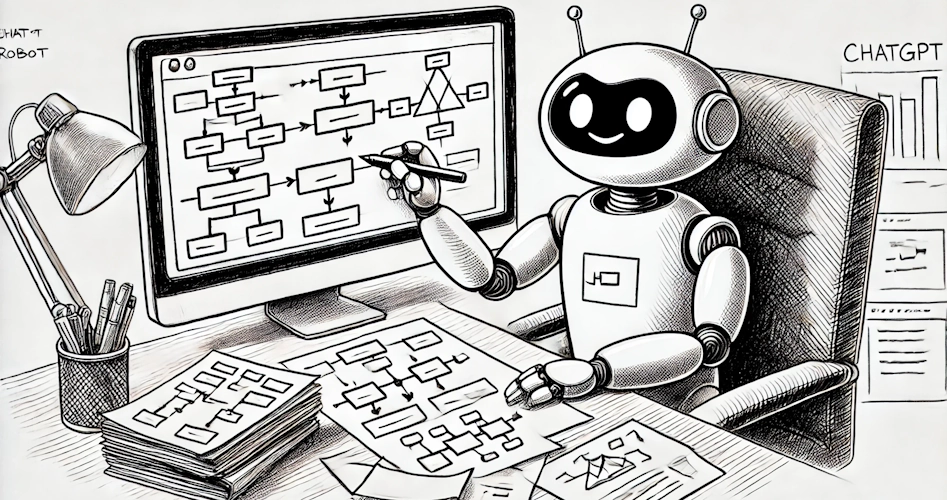
Creating architecture diagrams is a fundamental practice in software development and systems design. These diagrams help visualize complex systems, making it easier for teams to understand and communicate architectural ideas. Traditionally, this process can be time-consuming and requires a deep understanding of various diagramming tools.
However, with the advancements in AI, tools like ChatGPT can now assist in generating these diagrams quickly and accurately. By using natural language prompts, ChatGPT can produce diagram descriptions in formats compatible with popular tools like Draw.io, enhancing efficiency and collaboration.
This article will guide you through the steps to generate different types of architecture diagrams using ChatGPT, providing practical examples and tips to streamline your workflow. Whether you're a seasoned architect or a beginner, leveraging ChatGPT for diagram creation can significantly improve your productivity and the clarity of your designs.
Sequence Diagram
Sequence diagrams are a type of interaction diagram that shows how processes operate with one another and in what order. They are particularly useful for visualizing the sequence of messages between objects in a use case.
If you do not use text-to-diagram tools like Mermaid or PlantUML, and your company uses tools such as Draw.io for diagramming, creating a Sequence Diagram manually might be difficult and time-consuming.
Let's use ChatGPT to speed up the process:
✍️ Prompt to generate Sequence Diagram in ChatGPT:
Please generate a sequence diagram type to explain how DNS works. Provide results in mermaid format
🖺ChatGPT result:
sequenceDiagram
participant User
participant Browser
participant LocalDNSResolver
participant RootServer
participant TLDServer
participant AuthoritativeServer
User->>Browser: Enter example.com
Browser->>Browser: Check Cache
alt Cache Miss
Browser->>LocalDNSResolver: DNS Query for example.com
LocalDNSResolver->>LocalDNSResolver: Check Cache
alt Cache Miss
LocalDNSResolver->>RootServer: DNS Query for .
RootServer->>LocalDNSResolver: Respond with TLD Server (e.g., .com)
LocalDNSResolver->>TLDServer: DNS Query for example.com
TLDServer->>LocalDNSResolver: Respond with Authoritative Server
LocalDNSResolver->>AuthoritativeServer: DNS Query for example.com
AuthoritativeServer->>LocalDNSResolver: Respond with IP Address
end
LocalDNSResolver->>Browser: Return IP Address
end
Browser->>Browser: Cache IP Address
Browser->>example.com: HTTP Request
example.com->>Browser: HTTP Response
Converting to Draw.io Format:
1. Press Arrange > Insert > Advanced > Mermaid
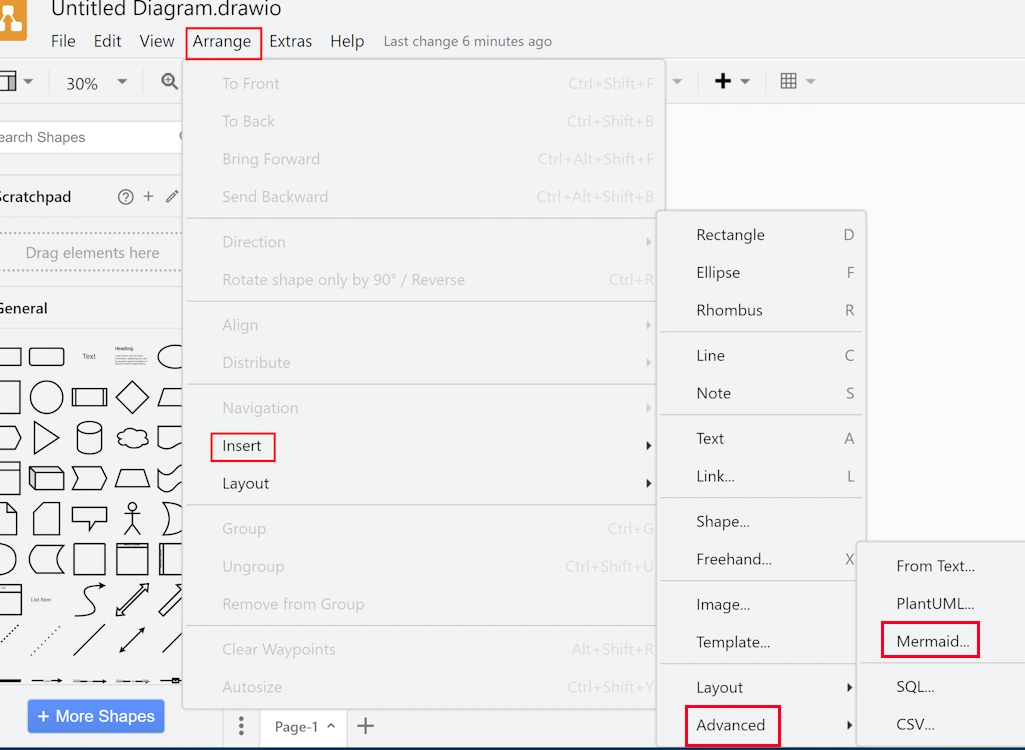
2. Paste the Mermaid text from ChatGPT output and press insert.
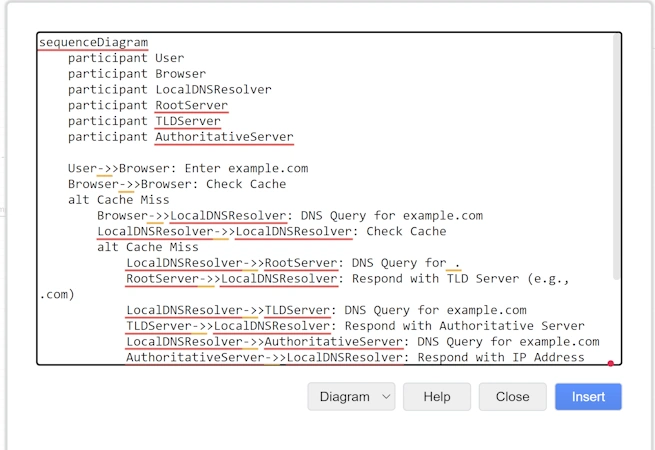
Here is the result:
Now you can customize styles or modify the flow as needed.
But you will ask, it's fine, but how can I generate something closer to my daily tasks? The same, you can add a prompt that will represent your needs, for example:
✍️ Prompt Example that is close to real-world example:
I need to generate a sequence diagram.
Here is my flow:
1) User send request into Orders service
2) Orders Service validate model
3) Orders service call Identity service to validate permissions
4) If identity service return not allowed, return forbidden to user
5) else if IF Identity service allowed, Order service call database to saving new order
6) Order service return to user success status
Provide result into mermaid format
🖺 Result:
sequenceDiagram
participant User
participant OrdersService
participant IdentityService
participant Database
User->>OrdersService: Send request
OrdersService->>OrdersService: Validate model
OrdersService->>IdentityService: Validate permissions
alt Not allowed
IdentityService-->>OrdersService: Return not allowed
OrdersService-->>User: Return forbidden
else Allowed
IdentityService-->>OrdersService: Return allowed
OrdersService->>Database: Save new order
Database-->>OrdersService: Return success
OrdersService-->>User: Return success status
end
Inserting into Draw.io:
Follow the same steps as above to insert the Mermaid text into Draw.io. (Press Arrange > Insert > Advanced > Mermaid)
Flowcharts
Modified Prompt for Flowchart:
✍️ ChatGPT Prompt to generate flowchart diagram:
I need to generate a flowchart diagram.
Here is my flow:
1) User send request into Orders service
2) Orders Service validate model
3) Orders service call Identity service to validate permissions
4) If identity service return not allowed, return forbidden to user
5) else if IF Identity service allowed, Order service call database to saving new order
6) Order service return to user success status
Provide result into mermaid format
🖺Result:
flowchart TD
A[User send request into Orders service] --> B[Orders Service validate model]
B --> C[Orders Service call Identity service to validate permissions]
C --> D{Identity service return allowed?}
D -->|No| E[Return forbidden to user]
D -->|Yes| F[Orders Service call database to save new order]
F --> G[Orders Service return to user success status]
Class Diagrams
Creating class diagrams from scratch can be time-consuming. Let's imagine we have the following .NET code base:
Order Model
namespace OrderManagement.Models
{
public class Order
{
public int Id { get; set; }
public string ProductName { get; set; }
public int Quantity { get; set; }
public decimal Price { get; set; }
}
}
IOrderRepository.cs
using OrderManagement.Models;
using System.Collections.Generic;
namespace OrderManagement.Repositories
{
public interface IOrderRepository
{
void AddOrder(Order order);
IEnumerable<Order> GetAllOrders();
Order GetOrderById(int id);
}
}
OrderRepository.cs
using OrderManagement.Models;
using System.Collections.Generic;
namespace OrderManagement.Repositories
{
public interface IOrderRepository
{
void AddOrder(Order order);
IEnumerable<Order> GetAllOrders();
Order GetOrderById(int id);
}
}
IOrderService.cs
using OrderManagement.Models;
using System.Collections.Generic;
namespace OrderManagement.Services
{
public interface IOrderService
{
void CreateOrder(Order order);
IEnumerable<Order> GetAllOrders();
Order GetOrderById(int id);
}
}
OrderService.cs
using OrderManagement.Models;
using OrderManagement.Repositories;
using System.Collections.Generic;
namespace OrderManagement.Services
{
public class OrderService : IOrderService
{
private readonly IOrderRepository _orderRepository;
public OrderService(IOrderRepository orderRepository)
{
_orderRepository = orderRepository;
}
public void CreateOrder(Order order)
{
_orderRepository.AddOrder(order);
}
public IEnumerable<Order> GetAllOrders()
{
return _orderRepository.GetAllOrders();
}
public Order GetOrderById(int id)
{
return _orderRepository.GetOrderById(id);
}
}
}
4. Order Controller
using Microsoft.AspNetCore.Mvc;
using OrderManagement.Models;
using OrderManagement.Services;
using System.Collections.Generic;
namespace OrderManagement.Controllers
{
[Route("api/[controller]")]
[ApiController]
public class OrderController : ControllerBase
{
private readonly IOrderService _orderService;
public OrderController(IOrderService orderService)
{
_orderService = orderService;
}
[HttpPost]
public IActionResult CreateOrder([FromBody] Order order)
{
_orderService.CreateOrder(order);
return Ok(order);
}
[HttpGet]
public ActionResult<IEnumerable<Order>> GetAllOrders()
{
var orders = _orderService.GetAllOrders();
return Ok(orders);
}
[HttpGet("{id}")]
public ActionResult<Order> GetOrderById(int id)
{
var order = _orderService.GetOrderById(id);
if (order == null)
{
return NotFound();
}
return Ok(order);
}
}
}
se
✍️ Prompt to generate class diagram:
can you generate mermaid class diagram based on code that I will provide below:
🖺 Result:
classDiagram
class Order {
int Id
string ProductName
int Quantity
decimal Price
}
class IOrderRepository {
<<interface>>
+ void AddOrder(Order order)
+ IEnumerable~Order~ GetAllOrders()
+ Order GetOrderById(int id)
}
class OrderRepository {
+ void AddOrder(Order order)
+ IEnumerable~Order~ GetAllOrders()
+ Order GetOrderById(int id)
}
class IOrderService {
<<interface>>
+ void CreateOrder(Order order)
+ IEnumerable~Order~ GetAllOrders()
+ Order GetOrderById(int id)
}
class OrderService {
- IOrderRepository _orderRepository
+ OrderService(IOrderRepository orderRepository)
+ void CreateOrder(Order order)
+ IEnumerable~Order~ GetAllOrders()
+ Order GetOrderById(int id)
}
class OrderController {
- IOrderService _orderService
+ OrderController(IOrderService orderService)
+ IActionResult CreateOrder(Order order)
+ ActionResult~IEnumerable~Order~~ GetAllOrders()
+ ActionResult~Order~ GetOrderById(int id)
}
OrderRepository ..|> IOrderRepository
OrderService ..|> IOrderService
OrderService --> IOrderRepository
OrderController --> IOrderService
Inserting into Draw.io:
Follow the same steps as above to insert the Mermaid text into Draw.io (Press Arrange > Insert > Advanced > Mermaid)
The full list of supported mermaid diagram types you can find by link.
C4 model diagrams
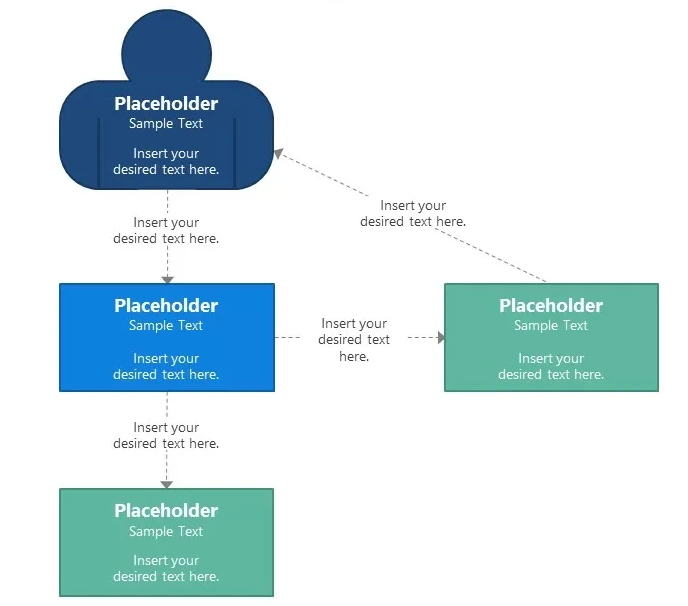
To generate a C4 model in a known format could be used, for example, structurizr DSL Language.
✍️ Prompt to generate C4 diagrams:
i have a system named MySuperSystem that connect to 3rd party to collect data by http.
Inside MySuperSystem i have 3 services. Parser that used to collect data from 3rd party by http.
Then parser send data to processor by gRPC and processor process data, thansform them and save into SQL DB.
Then API collect data from db and present it to user wirth role manager. Could you generate c4 diagram using structurizr DSL Language
🖺 Result:
workspace {
model {
user = person "Manager" {
description "User who manage collected data"
}
thirdParty = softwareSystem "Third Party System" {
description "External system providing data via HTTP"
}
mySuperSystem = softwareSystem "MySuperSystem" {
description "A system that collects data from a third party, processes it, and presents it to the user"
webBrowser = container "Web Browser" {
description "Allows the user to interact with MySuperSystem"
technology "JavaScript/HTML/CSS"
}
api = container "API" {
description "Provides endpoints for the web application to interact with"
technology "Spring Boot"
}
sqlDatabase = container "SQL Database" {
description "Stores processed data"
technology "PostgreSQL"
}
processor = container "Processor" {
description "Processes and transforms data received from the parser"
technology "gRPC Service"
}
parser = container "Parser" {
description "Collects data from a third party"
technology "HTTP Client"
}
user -> webBrowser "Uses"
webBrowser -> api "Sends requests to"
api -> sqlDatabase "Queries data from"
parser -> thirdParty "Fetches data from" "HTTP"
parser -> processor "Sends data to" "gRPC"
processor -> sqlDatabase "Stores processed data in"
api -> processor "Sends request to process data"
api -> sqlDatabase "Fetches data from"
}
}
views {
systemContext mySuperSystem {
include *
autolayout lr
title "System Context diagram for MySuperSystem"
}
container mySuperSystem {
include *
autolayout lr
title "Container diagram for MySuperSystem"
}
theme default
}
}
Then paste the result into structurizr. Now you can adjust diagrams to your needs and download the diagrams.
Here is an example of a System context diagram.
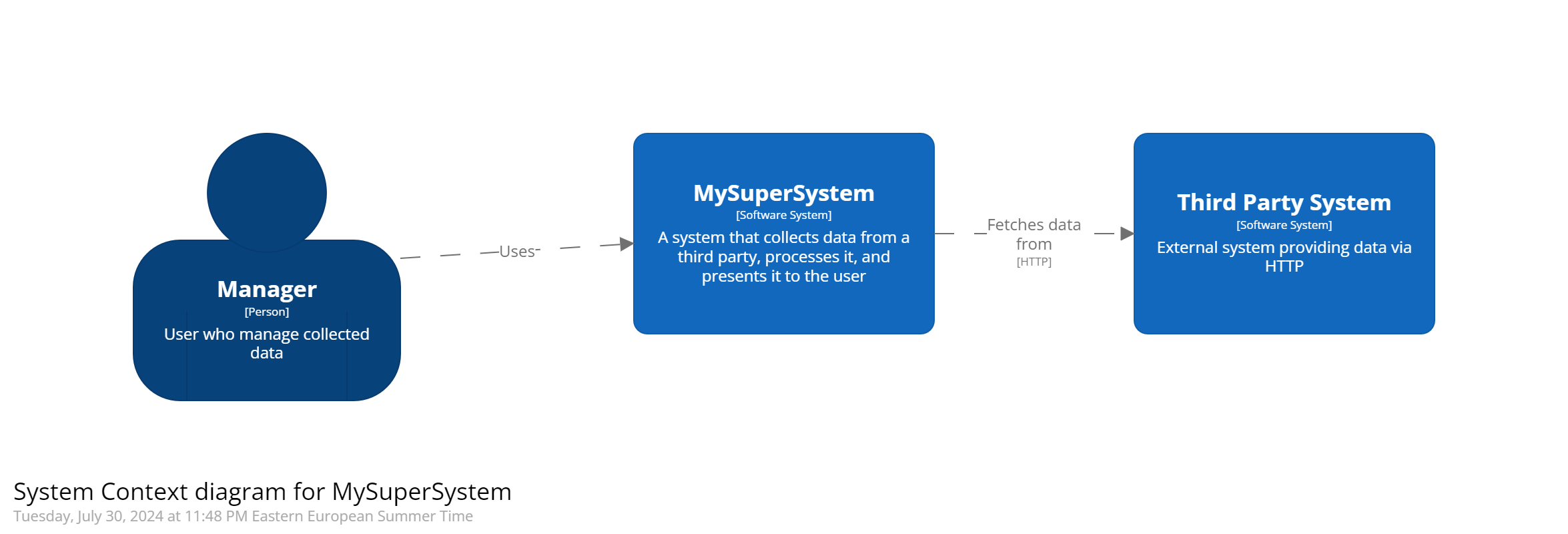
An example of a Container diagram
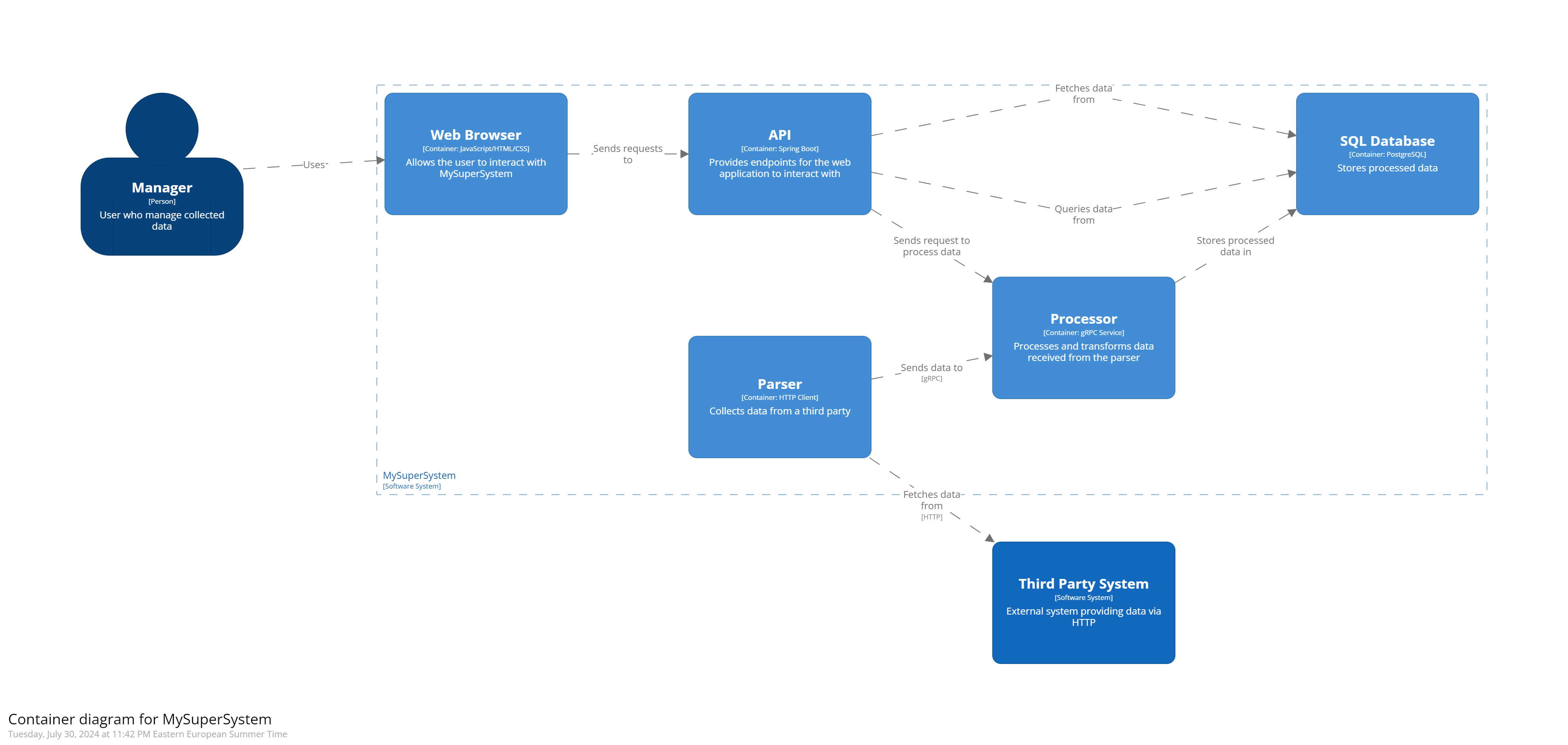
Deployment diagram
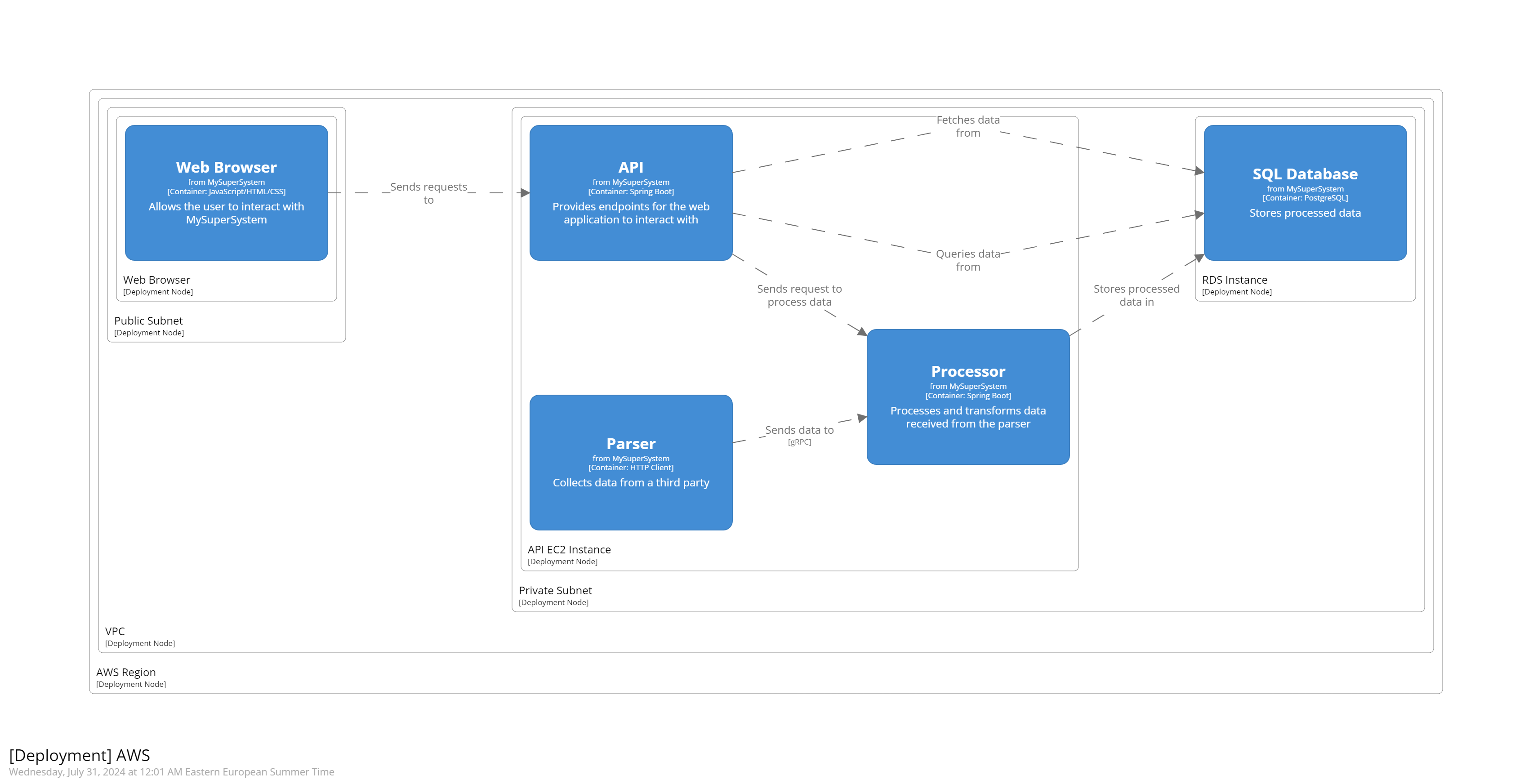
Then, when we have a container we need to make a deployment diagram for it, I'ts might be difficult, as we can't have access to our DevOps at the moment and research could take significant time. So our co-pilot ChatGPT can help us to generate a deployment diagram. Let's reuse our container diagram for generating a deployment diagram in AWS.
Note: Of course, this diagram cannot be used 'as is'. But it could be a starting point that you can modify to your needs.
✍️ Prompt to generate C4 Deployment diagram:
could you generate deployment diagram using structurizr for AWS via following diagram
workspace {
model {
user = person "Manager" {
description "User who manage collected data"
}
thirdParty = softwareSystem "Third Party System" {
description "External system providing data via HTTP"
}
mySuperSystem = softwareSystem "MySuperSystem" {
description "A system that collects data from a third party, processes it, and presents it to the user"
webBrowser = container "Web Browser" {
description "Allows the user to interact with MySuperSystem"
technology "JavaScript/HTML/CSS"
}
api = container "API" {
description "Provides endpoints for the web application to interact with"
technology "Spring Boot"
}
sqlDatabase = container "SQL Database" {
description "Stores processed data"
technology "PostgreSQL"
}
processor = container "Processor" {
description "Processes and transforms data received from the parser"
technology "Spring Boot"
}
parser = container "Parser" {
description "Collects data from a third party"
technology "HTTP Client"
}
user -> webBrowser "Uses"
webBrowser -> api "Sends requests to"
api -> sqlDatabase "Queries data from"
parser -> thirdParty "Fetches data from" "HTTP"
parser -> processor "Sends data to" "gRPC"
processor -> sqlDatabase "Stores processed data in"
api -> processor "Sends request to process data"
api -> sqlDatabase "Fetches data from"
}
}
views {
systemContext mySuperSystem {
include *
autolayout lr
title "System Context diagram for MySuperSystem"
}
container mySuperSystem {
include *
autolayout lr
title "Container diagram for MySuperSystem"
}
theme default
}
}
🖺Result:
workspace {
model {
user = person "Manager" {
description "User who manages collected data"
}
thirdParty = softwareSystem "Third Party System" {
description "External system providing data via HTTP"
}
mySuperSystem = softwareSystem "MySuperSystem" {
description "A system that collects data from a third party, processes it, and presents it to the user"
webBrowser = container "Web Browser" {
description "Allows the user to interact with MySuperSystem"
technology "JavaScript/HTML/CSS"
}
api = container "API" {
description "Provides endpoints for the web application to interact with"
technology "Spring Boot"
}
sqlDatabase = container "SQL Database" {
description "Stores processed data"
technology "PostgreSQL"
}
processor = container "Processor" {
description "Processes and transforms data received from the parser"
technology "Spring Boot"
}
parser = container "Parser" {
description "Collects data from a third party"
technology "HTTP Client"
}
user -> webBrowser "Uses"
webBrowser -> api "Sends requests to"
api -> sqlDatabase "Queries data from"
parser -> thirdParty "Fetches data from" "HTTP"
parser -> processor "Sends data to" "gRPC"
processor -> sqlDatabase "Stores processed data in"
api -> processor "Sends request to process data"
api -> sqlDatabase "Fetches data from"
}
development = deploymentEnvironment "AWS" {
deploymentNode "AWS Region" {
deploymentNode "VPC" {
deploymentNode "Public Subnet" {
deploymentNode "Web Browser" {
containerInstance webBrowser
}
}
deploymentNode "Private Subnet" {
deploymentNode "API EC2 Instance" {
containerInstance api
containerInstance processor
containerInstance parser
}
deploymentNode "RDS Instance" {
containerInstance sqlDatabase
}
}
}
}
}
}
views {
systemContext mySuperSystem {
include *
autolayout lr
title "System Context diagram for MySuperSystem"
}
container mySuperSystem {
include *
autolayout lr
title "Container diagram for MySuperSystem"
}
deployment * development {
include *
autoLayout lr
}
theme default
}
}
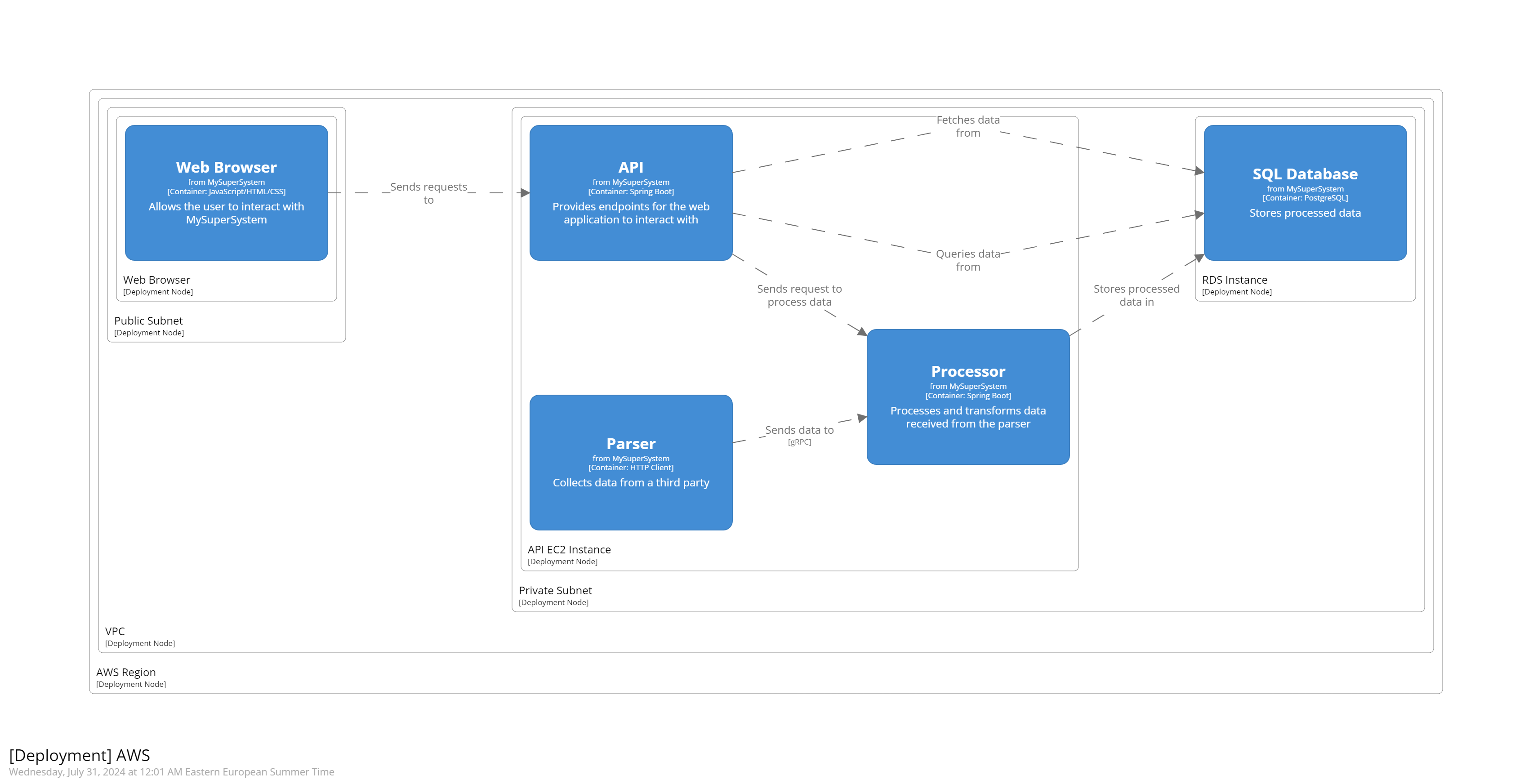
ChatGPT Show Me Plugin
If you have a paid version of ChatGPT, use used Show me GPT plugin. It uses PlainUML and Miro to generate diagrams for you.
For example, let's ask the plugin to explain how DNS works:
✍️ Prompt:
show me how dns works
🖺 Result:
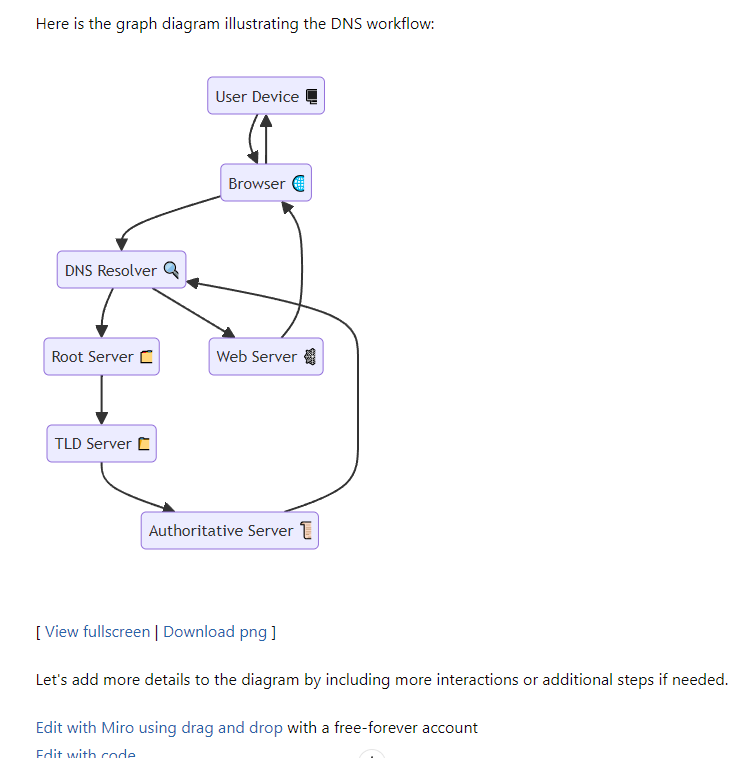
The plugin generates a diagram in mermaid format and a Miro page that you can use to edit styles, etc.
Conclusion
Utilizing ChatGPT for generating architecture diagrams revolutionizes the traditional approach by making it faster and more efficient. This method allows you to create detailed and accurate diagrams through natural language prompts, seamlessly integrating with tools like Draw.io. This not only saves time but also improves collaboration and clarity in your design processes.
By following the steps outlined in this article, you can harness the power of AI to enhance your workflow, ensuring that your architectural visions are clearly communicated and easily understood by your team. Experiment with different prompts and refine your diagrams to meet your specific needs, transforming the way you approach system design and documentation.