Top 50+ NuGet packages для .NET разработчика
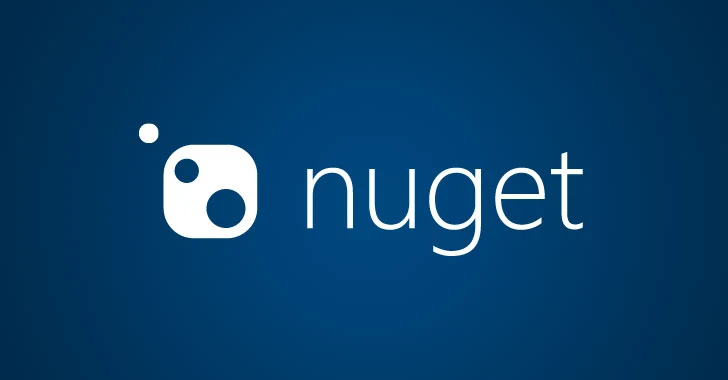
В этой статье собрали лучшие nuget пакеты для .NET разработки, которые смогут вам пригодится в повседневной разработке
Mapping

Для инкапсуляции домена, мы часто юзаем DTO для наших объектов и маппить все проперти entity вручную так себе идея, для этого существуют библиотеки, которые делают это за вас, рассмотрим самые популярные из них:
⭐Riok.Mapperly
Поскольку Mapperly создает код сопоставления во время сборки, во время выполнения накладные расходы минимальны. Следовательно - это довольно производительная либа.
- Mapperly не использует reflection
- Mapperly безопасен для обрезки и AoT
- Mapperly запускается во время сборки
- Mapperly не имеет зависимостей во время выполнения
- Генерируемые маппинги быстры с минимальными затратами памяти
- Сгенерированный код маппинга читаем и отлаживаем
- Нет необходимости писать и поддерживать boilerplate вручную
- Mapperly является подключаемым: всегда можно реализовать маппинги для определенных типов вручную, которые будут подхвачены Mapperly
Пример как это работает, можно подсмотреть в их документации.
⭐Mapster
- Простота использования, высокая скорость и низкое потребление памяти.
- Для использования Mapster нам не нужно создавать какую-либо предварительную конфигурацию. Он работает через методы расширения, поэтому маппить entity на новый экземпляр другого класса очень просто.
AutoMapper
Самая популярная либа, но не самый производительный варик для маппинга
Logging

Куда ж без логов, вот несколько самых популярных библиотек .NET для логирования
⭐Serilog
Позволяет писать structrured log by default. В остальном тоже ничем не уступает аналогам.
Пример как работает лог:
var position = new { Latitude = 25, Longitude = 134 };
var elapsedMs = 34;
log.Information("Processed {@Position} in {Elapsed:000} ms.", position, elapsedMs);
В результате получаем что-то типа
:14:22 [Information] Processed { Latitude: 25, Longitude: 134 } in 034 ms.
Можно писать логи в:
- Консоль
- Файл
- БД
- GrayLog
и многое другое.
NLog
NLog предлагает систему конфигурации, позволяющую детально настраивать логирование. Оптимизирован для высокой производительности и низкого потребления ресурсов.
log4net
log4net - проверенное временем решение, которое используется во многих проектах. Поддерживает конфигурацию через файлы конфигурации, так и программно.
Разработка log4net не так активна, как у некоторых других библиотек для логов.
Microsoft.Extensions.Logging
Плотно интегрирована с .NET Core и ASP.NET Core, что обеспечивает легкую настройку и использование. Позволяет легко переключаться между различными провайдерами логирования без изменения кода приложения.
Меньше функциональности по сравнению со специализированными библиотеками: Как абстракция, может не предоставлять некоторые продвинутые возможности, доступные в таких библиотеках, как NLog или log4net.
RDBMS и ORM'ки

Для работы с реалиционными БД есть много разных либ, конечно же самые популярные это ORM EF и Dapper. DbUp довольно мощная тула для миграции БД.
⭐Entity Framework Core
Это ORM фреймворк от Microsoft, предназначенный для работы с базами данных в .NET приложениях. Он позволяет разработчикам работать с данными через объекты .NET, минимизируя необходимость написания SQL кода. Entity Framework Core поддерживает миграции баз данных, ленивую загрузку и автоматическое отслеживание изменений в объектах, что упрощает разработку.
⭐Dapper
Dapper — легковесный ORM фреймворк, который предоставляет минимальную абстракцию над SQL. Он разработан командой StackOverflow и ориентирован на производительность и простоту. Dapper позволяет напрямую работать с SQL запросами, обеспечивая высокую скорость выполнения за счет отказа от сложных функций, таких как автоматическое отслеживание изменений или ленивая загрузка, доступных в более тяжеловесных ORM, таких, как EF.
⭐DbUp
DbUp - набор библиотек .NET, которые помогают развертывать изменения в различных базах данных, таких как SQL Server. Он отслеживает, какие SQL миграции уже были запущены, и запускает те, которые необходимы для обновления базы данных. По сути, работает по принципу миграции в EF, но если вы юзаете Dapper или вовсе без ORM, то DbUp будет полезным.
NoSQL

Куда ж без NoSQL, вот самые популярные NoSQL решения и nuget пакеты для них:
MongoDB Driver
Либы для работы с MongoDB из .NET приложений. Подробнее документацию можно посмотреть на официальном сайте.
AWS DynamoDB
Этот пакет предоставляет удобный доступ к DynamoDB, позволяя эффективно работать с данными в этой NoSQL базе данных, включая операции создания, чтения, обновления и удаления данных, а также поддержку различных моделей данных.
RavenDB Client
RavenDB Client — библиотека для взаимодействия с RavenDB, NoSQL базой данных.
Azure Cosmos DB
Либа для работы с CosmosDB от Azure
Marten
Marten — библиотека для .NET, которая позволяет использовать PostgreSQL как документо-ориентированную базу данных. Она предлагает легкую работу с документами JSON прямо в PostgreSQL, обеспечивая возможности такие как LINQ-запросы, полнотекстовый поиск и версионирование документов.
HTTP

Довольно часто приходится общаться с внешними API, эти библиотеки помогут вам в этом
⭐Polly
Polly — библиотека для .NET, предназначенная для обработки повторных попыток, Circuit Breaker и других стратегий устойчивости к сбоям. Она позволяет разработчикам легко настраивать политики для повторных попыток, тайм-аутов, обработки исключений и многого другого, что делает приложения более надежными при возникновении ошибок во внешних вызовах или временных сбоях.
⭐RestSharp
RestSharp — это простой и гибкий HTTP-клиент для .NET, который облегчает взаимодействие с RESTful API. Поддерживает асинхронные вызовы и автоматическую десериализацию ответов
Refit
Refit — библиотека REST клиента для .NET, которая превращает ваш HTTP API в живой .NET интерфейс. Вместо ручного создания HttpClient вызовов, Refit позволяет определить интерфейс с методами и аннотациями, соответствующими вашему API. Это упрощает код, делая его более читаемым и лаконичным.
Встроенная HttpFactory
Валидации

Ни 1 .NET проект не обходится без валидации данных на стороне сервера, ниже рассмотрим популярные либы для этого:
⭐FluentValidation
FluentValidation — библиотека для .NET, предназначенная для создания мощных валидационных правил с использованием легко читаемого, почти естественного языка. Она позволяет разработчикам определять правила валидации в отдельных классах валидаторов, к примеру:
using FluentValidation;
public class CustomerValidator: AbstractValidator<Customer> {
public CustomerValidator() {
RuleFor(x => x.Surname).NotEmpty();
RuleFor(x => x.Forename).NotEmpty().WithMessage("Please specify a first name");
RuleFor(x => x.Discount).NotEqual(0).When(x => x.HasDiscount);
RuleFor(x => x.Address).Length(20, 250);
RuleFor(x => x.Postcode).Must(BeAValidPostcode).WithMessage("Please specify a valid postcode");
}
private bool BeAValidPostcode(string postcode) {
// custom postcode validating logic goes here
}
}
var customer = new Customer();
var validator = new CustomerValidator();
// Execute the validator
ValidationResult results = validator.Validate(customer);
// Inspect any validation failures.
bool success = results.IsValid;
List<ValidationFailure> failures = results.Errors;
DataAnnotations
DataAnnotations — это атрибуты, встроенные в .NET, которые можно применять непосредственно к классам или свойствам для указания правил валидации, метаданных или других инструкций, используемых фреймворками, такими как Entity Framework и ASP.NET MVC. DataAnnotations обеспечивают простой способ включения базовой валидации и метаданных непосредственно в модели данных вашего приложения. Хотя они менее гибкие по сравнению с FluentValidation, DataAnnotations предлагают простой и быстрый способ добавления валидации без необходимости создания отдельных классов валидаторов.
Auth

⭐Microsoft.Identity
Microsoft.Identity включает в себя библиотеки, такие как Microsoft.Identity.Client (MSAL) и Microsoft.AspNetCore.Identity, предназначенные для управления пользователями, аутентификации и авторизации в .NET приложениях. MSAL упрощает интеграцию с Microsoft Identity Platform (включая Azure AD и Microsoft accounts), обеспечивая доступ к токенам безопасности для вызова защищенных API. AspNetCore.Identity предлагает API для управления пользователями, паролями, профилями, ролями и т.д. в веб-приложениях.

Так же с .NET 8 набирает популярность подход с Identity Endpoints.
Identity Server / Duende Identity Server

Identity Server (ныне известный как Duende Identity Server после коммерциализации) — это фреймворк OpenID Connect и OAuth 2.0 для ASP.NET Core, который позволяет реализовать собственный сервер аутентификации и авторизации. Это решение широко используется для создания систем безопасности и идентификации, поддерживающих современные стандарты и обеспечивающих защиту API.
И много других библиотек в зависимости от ваших нужд...
Для более комплексных кейсов стоит рассмотреть готовые решения такие как:
Тестирование

⭐NUnit
Популярный фреймворк для модульного тестирования в .NET, поддерживающий много функций для создания комплексных тестовых сценариев.
⭐xUnit
Еще один современный фреймворк для модульного тестирования, разработанный с учетом новых возможностей .NET. Он предлагает улучшенную модель тестирования по сравнению с NUnit и MSTest, включая поддержку параллельного выполнения тестов и уникальную систему фикстур.
⭐FluentAssertions
Предоставляет набор методов расширения который улучшает читаемость кода и упрощает работу с Assets.
FakeItEasy
Библиотека для создания фейков (заглушек и моков) в .NET, позволяющая легко подменять зависимости в тестируемом коде. Она предлагает простой и интуитивно понятный API для настройки поведения фейков.
Bogus
Либа для генерации фиктивных данных в .NET. Она позволяет легко создавать тестовые данные для модульных тестов, заглушек или демонстрационных приложений, поддерживая широкий спектр типов данных.
WireMock.Net
Предоставляет возможность имитации HTTP-серверов для тестирования взаимодействия с веб сервисами. Это полезно для тестирования клиентского кода без необходимости обращения к реальным веб сервисам, что ускоряет тестирование и делает его более надежным.
Moq
Библиотека для в .NET, которая позволяет создавать моки для интерфейсов и классов.
NSubstitute
Библиотека для создания моков и заглушек с простым API, ориентированная на удобство и простоту использования. Она позволяет настраивать возвращаемые значения и проверять вызовы методов без необходимости написания большого количества шаблонного кода.
AutoFixture
Библиотека, которая автоматизирует настройку тестовых данных для модульных тестов, создавая объекты с заполненными данными автоматически. Это снижает количество необходимого ручного кода и упрощает подготовку данных для тестов.
⭐SpecFlow.NUnit
SpecFlow.NUnit интегрирует фреймворк BDD (Behavior-Driven Development) SpecFlow с NUnit, позволяя разработчикам определять тесты через спецификации на естественном языке.
Task Schedulers

⭐Quartz.NET
Quartz.NET — это полнофункциональный планировщик задач для .NET приложений, который позволяет разработчикам запускать задачи по расписанию. Он поддерживает простые и сложные сценарии планирования, включая возможность использования CRON-выражений. Quartz.NET может быть использован для добавления сложных планировщиков задач в любое .NET приложение.
⭐Hangfire
Hangfire — это фреймворк для фонового выполнения задач в .NET приложениях, который позволяет планировать и выполнять задачи асинхронно на фоне. Он предлагает удобный дашборд для мониторинга и управления фоновыми задачами, поддерживает персистентность задач и не требует отдельного сервиса для управления очередями задач.
FluentScheduler
FluentScheduler — это библиотека для автоматизации задач в .NET, которая позволяет легко планировать выполнение задач с использованием флюент API. Она поддерживает одноразовые и повторяющиеся задачи, а также предоставляет возможность организации задач в группы для удобства управления.
NCrontab
Библиотека, предоставляющая парсер и форматтер для CRON-выражений в .NET. Хотя сама по себе не является планировщиком задач, она может быть использована в сочетании с другими инструментами для расширения возможностей планирования задач с использованием стандартного CRON-формата.
Messaging

RabbitMQ.Client
Библиотека для работы с RabbitMQ
Kafka
Библиотека для работы с Kafka
Confluent.Kafka
Confluent.Kafka это .NET клиент для Apache Kafka, разработанный Confluent. Эта библиотека предоставляет производительный и удобный API для паблиша и получения сообщений из Kafka. Confluent.Kafka тесно интегрирована с Kafka и поддерживает все основные возможности Kafka, включая транзакции, потоковую обработку и управление схемами через Confluent Schema Registry,
MassTransit
это легковесная библиотека для .NET, облегчающая работу с брокерами сообщений, такими как RabbitMQ и Kafka. Она предоставляет высокоуровневый API для асинхронного взаимодействия между компонентами приложения через сообщения. MassTransit упрощает реализацию сложных шаблонов обмена сообщениями и обеспечивает интеграцию с системами контроля версий сообщений, компенсационными транзакциями и мониторингом.
Кеширование

⭐ZiggyCreatures.FusionCache
Довольно мощьная либа, поддерживает как inmemory так и distributed кэш. Моя персональная рекомендация.
Microsoft.Extensions.Caching
Microsoft.Extensions.Caching включает в себя две основные реализации кэша: MemoryCache для кэширования в памяти и DistributedCache для распределенного кэширования.
Другое
⭐MediatR
Библиотека, реализующая шаблон "Посредник", который помогает уменьшить прямую зависимость между компонентами программы, облегчая тем самым их тестирование и поддержку.
⭐Newtonsoft.Json
Популярная библиотека для работы с Json.
⭐System.Text.Json
Альтернатива Newtonsoft.Json для работы с Json от Microsoft.
GraphQL
Библиотека для работы с GraphQL.
Пишите в комментах, чего не хватает и что еще следует добавить